This is a library to create AlertDialog , ProgressDialog and etc...
Click
▶️ or 🔽 to expand or collapse examples
AlertDialog
var alert = new AlertDialog.Builder()
.setDialogTitle("Title")
.setDialogMessage("Message")
.setPositiveButton("Ok", System.out::println)
.create();
alert.setTitle(alert.getDialogTitle());
alert.show();
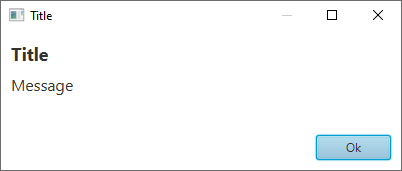
MultiChoiceAlertDialog
var alert = new AlertDialog.Builder()
.setDialogTitle("Title")
.setDialogMessage("Message")
.setPositiveButton("Ok", System.out::println)
.setMultiChoiceItems(new String[]{"A","B","C","D","E"}, new Integer[]{0,2,3}, (which, isChecked) -> {
if (isChecked)
System.out.println(which + " checked");
})
.create();
alert.setTitle(alert.getDialogTitle());
alert.show();
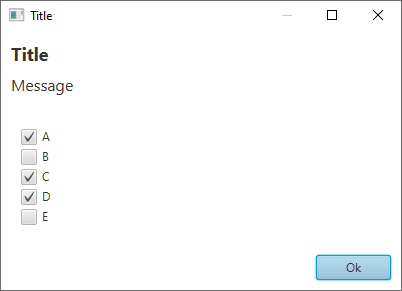
SingleChoiceAlertDialog
var alert = new AlertDialog.Builder()
.setDialogTitle("Title")
.setDialogMessage("Message")
.setPositiveButton("Ok", System.out::println)
.setSingleChoiceItems(new String[]{"A","B","C","D"}, 2, which -> System.out.println(which + "checked"))
.create();
alert.setTitle(alert.getDialogTitle());
alert.show();
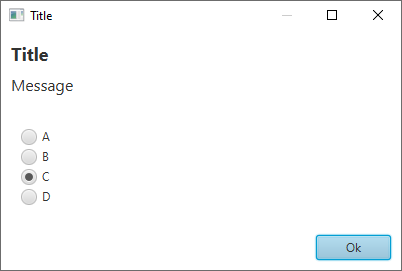
CustomAlertDialog
var customNodeContainer = new HBox();
customNodeContainer.setPadding(new Insets(10));
var textField = new TextField();
HBox.setHgrow(textField, Priority.ALWAYS);
textField.setPromptText("Name");
customNodeContainer.getChildren().add(textField);
var alert = new AlertDialog.Builder()
.setDialogTitle("Name")
.setDialogMessage("Enter your name in below text field")
.setPositiveButton("Ok", which -> System.out.println(textField.getText()))
.setNode(customNodeContainer)
.create();
alert.setTitle(alert.getDialogTitle());
alert.show();
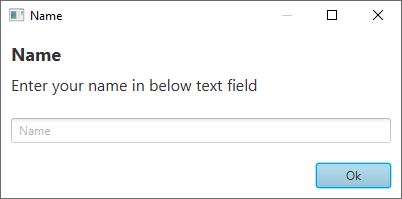
AlertDialogWithSound
var alert = new AlertDialog.Builder()
.setDialogTitle("DialogWithSound")
.setDialogMessage("The sound will be play when dialog shown")
.setPositiveButton("Ok", System.out::println)
.setSound(Sounds.ChimesGlassy)
.create();
alert.setTitle(alert.getDialogTitle());
alert.show();
Note : you should add javafx.media dependency when you want to use sounds
TimePickerDialog
var timePickerDialog = new TimePickerDialog.Builder()
.create();
timePickerDialog.initModality(Modality.APPLICATION_MODAL);
timePickerDialog.showAndWait();
System.out.println(timePickerDialog.getTime().toString());
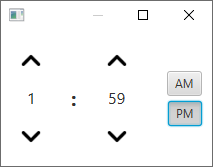
ProgressDialog
var progressDialog = new ProgressDialog.Builder()
.setDialogTitle("Title")
.setDialogMessage("Message")
.setProgressType(ProgressDialog.ProgressBarType.Bar)
.setProgress(.5)
.create();
progressDialog.setTitle(progressDialog.getDialogTitle());
progressDialog.show();
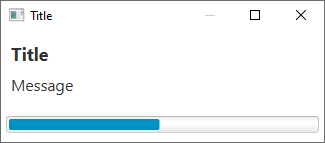
ExceptionDialog
try {
System.out.println(20/0);
}catch (ArithmeticException e) {
var exceptionDialog = new ExceptionDialog.Builder()
.setDialogMessage(e.getMessage())
.setException(e)
.create();
exceptionDialog.show();
}
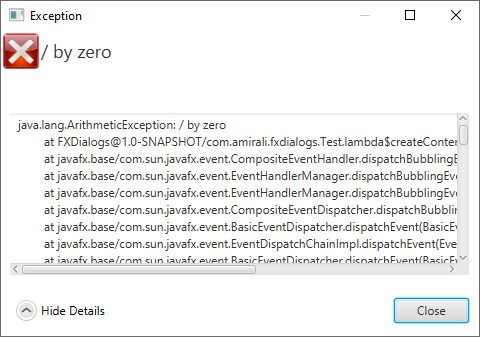
PersistentBottomSheet
var persistentBottomSheet = new PersistentBottomSheet();
persistentBottomSheet.setPrefHeight(150);
persistentBottomSheet.setStyle("-fx-background-color : orange;");
persistentBottomSheet.setDuration(Duration.seconds(.5));
persistentBottomSheet.addResizingSupport();
var label = new Label("Bottom Sheet");
label.setStyle("-fx-text-fill : white; -fx-font-size : 18px;");
var bottomSheetContentRoot = new StackPane(label);
bottomSheetContentRoot.setPadding(new Insets(15));
persistentBottomSheet.getChildren().add(bottomSheetContentRoot);
var showButton = new Button("Hide");
showButton.setPrefSize(75, 25);
showButton.setOnAction(event -> {
persistentBottomSheet.setShowing(!persistentBottomSheet.isShowing());
if (persistentBottomSheet.isShowing())
showButton.setText("Hide");
else
showButton.setText("Show");
});
var region = new Region();
region.setMaxHeight(Double.MAX_VALUE);
VBox.setVgrow(region, Priority.ALWAYS);
var root = new VBox(showButton, region, persistentBottomSheet);
root.setAlignment(Pos.CENTER);
BottomSheetCallBack
persistentBottomSheet.setCallBack(new BottomSheetCallBack() {
@Override
public void onState(PersistentBottomSheet bottomSheet, int state) {
switch (state) {
case PersistentBottomSheet.EXPANDING ->
System.out.println("expanding");
case PersistentBottomSheet.COLLAPSING ->
System.out.println("collapsing");
case PersistentBottomSheet.DRAGGED ->
System.out.println("dragged");
case PersistentBottomSheet.HIDDEN ->
System.out.println("hidden");
case PersistentBottomSheet.SHOWN ->
System.out.println("shown");
}
}
@Override
public void onResized(PersistentBottomSheet bottomSheet, double height) {
System.out.println(height);
}
});
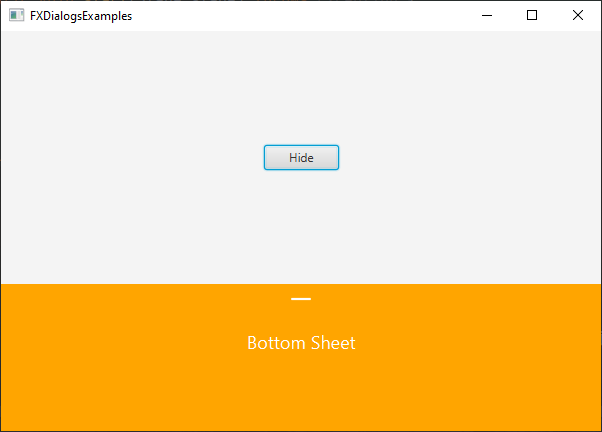
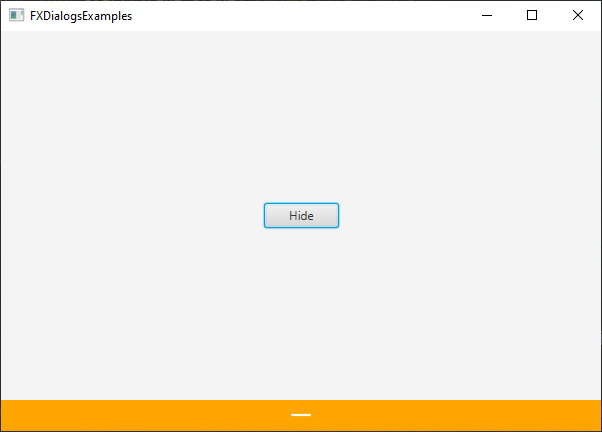
SplashScreen
import com.amirali.fxdialogs.SplashScreen;
import javafx.application.Application;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
import javafx.util.Duration;
public class App extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("SplashScreenDemo");
primaryStage.setScene(new Scene(createContent(), 600, 400));
var splashScreen = new SplashScreen.Builder(Duration.seconds(2), primaryStage)
.setLayout(createSplashScreenContent())
.create();
splashScreen.show();
}
private Parent createSplashScreenContent() {
var label = new Label("SplashScreenDemo");
label.setStyle("-fx-font-size: 25px; -fx-font-weight: bold; -fx-text-fill: white;");
var root = new StackPane(label);
root.setStyle("-fx-background-color: linear-gradient(to right, #FF508E, #5AC0FF);");
root.setPrefSize(600, 400);
return root;
}
private Parent createContent() {
var label = new Label("Hello, World");
label.setStyle("-fx-font-size: 20px;");
var root = new StackPane(label);
root.setStyle("-fx-background-color: white;");
return root;
}
}
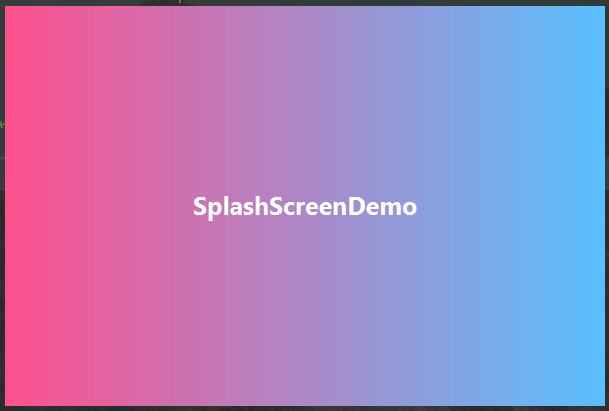
after 2 seconds
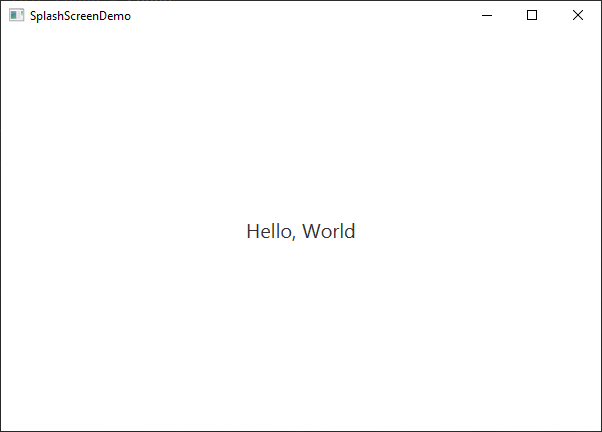
PopupNotification
- create notification layout (of course you can set controller)
notification-view.fxml
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.control.Label?>
<?import javafx.scene.layout.AnchorPane?>
<AnchorPane styleClass="notification" prefHeight="150.0" prefWidth="320.0" stylesheets="@style.css" xmlns="http://javafx.com/javafx/17" xmlns:fx="http://javafx.com/fxml/1">
<children>
<Label styleClass="title" fx:id="title" layoutX="14.0" layoutY="14.0" text="ExampleNotification" AnchorPane.leftAnchor="0.0" AnchorPane.rightAnchor="0.0" AnchorPane.topAnchor="0.0" />
<Label styleClass="message" fx:id="message" layoutX="8.0" layoutY="46.0" text="This is a custom notification" wrapText="true" AnchorPane.leftAnchor="0.0" AnchorPane.rightAnchor="0.0" />
</children>
</AnchorPane>
- style sheet
style.css
.notification {
-fx-background-color: white;
-fx-background-radius: 5px;
-fx-background-insets: 5px;
-fx-effect: dropshadow(three-pass-box, black, 10, 0, 0, 0);
-fx-padding: 8px;
}
.notification .title {
-fx-font-size: 18px;
-fx-font-weight: bold;
}
.notification .message {
-fx-font-size: 16px;
-fx-alignment: top-left;
}
- create and show the notification
try {
var loader = new FXMLLoader(getClass().getResource("notification-view.fxml"));
var notification = new PopupNotification(Duration.seconds(2), ((AnchorPane) loader.load()));
notification.setSound(Sounds.Succeeded);
// be sure to always choose a unique id for every notification
notification.setNotificationId(UUID.randomUUID().toString());
notification.show(primaryStage);
}catch (IOException e) {
e.printStackTrace();
}
result :
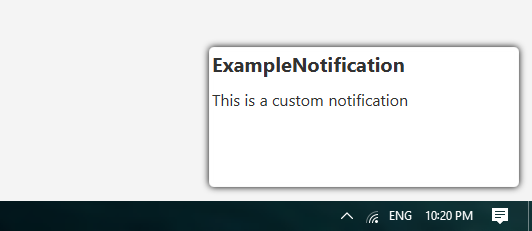
TrayIcon
if (SystemTray.isSupported()) {
Platform.setImplicitExit(false);
var menu = new ContextMenu();
var exitItem = new MenuItem("Exit Application");
exitItem.setOnAction(event -> {
Platform.exit();
System.exit(0);
});
menu.getItems().add(exitItem);
var trayIcon = new FXTrayIcon(
Toolkit.getDefaultToolkit().getImage(getClass().getResource("fxdialogs.png")),
"Demo FXTrayIcon",
menu
);
var tray = SystemTray.getSystemTray();
tray.add(trayIcon);
}
result:
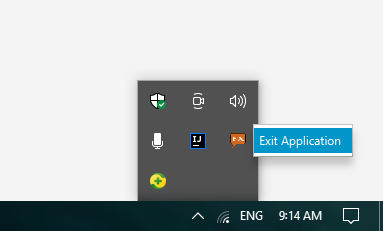
SimplePopupNotification
var notification = new SimplePopupNotification(Duration.seconds(2), "SimplePopupNotification", "This is a simple popup notification");
// be sure to always choose a unique id for every notification
notification.setNotificationId(UUID.randomUUID().toString());
notification.show(primaryStage);
style.css
.simple-notification {
-fx-background-color: white;
-fx-background-radius: 5px;
-fx-background-insets: 5px;
-fx-effect: dropshadow(three-pass-box, black, 10, 0, 0, 0);
-fx-padding: 8px;
}
.simple-notification .title {
-fx-font-size: 18px;
-fx-font-weight: bold;
}
.simple-notification .message {
-fx-font-size: 16px;
-fx-alignment: top-left;
}
.simple-notification .close {
-fx-background-color: transparent;
}
.simple-notification .close:hover {
-fx-background-color: #9E9E9E;
}
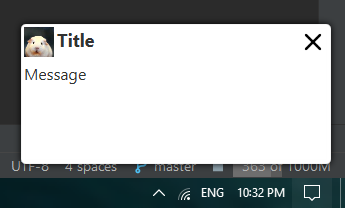
example for alert dialog :
.alert-dialog {
-fx-background-color : white;
}
.alert-dialog .title {
-fx-font-size : 18px;
-fx-font-weight : bold;
}
.alert-dialog .message {
-fx-font-size : 16px;
}