This repository consists of solutions to HackerRank practice, tutorials, and interview preparation problems with Python, mySQL, C#, and JavaScript.
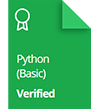
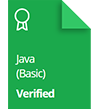
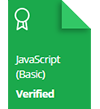
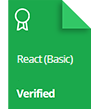
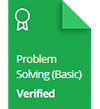
💻 This is my approach of solving the questions of
- 30 Days of Code on Hackerrank ✔️
- C Language ✔️
- Java (Language Proficiency) ✔️
- Problem Solving ✔️
- SQL ✔️
Hackerrank : https://www.hackerrank.com/ashishkumar_cse2
Linkedin in : www.linkedin.com/in/ashish-kumar-2030
Github : https://github.com/Ashish2030
Subdomain | Challenge | Problem | Difficulty | Score | Solution |
---|---|---|---|---|---|
Introduction | Say 'Hello, World!' With Python | Problem | Easy | 5 | Solution |
Introduction | Python If-Else | Problem | Easy | 10 | Solution |
Introduction | Arithmetic Operators | Problem | Easy | 10 | Solution |
Introduction | Python Division | Problem | Easy | 10 | Solution |
Introduction | Loops | Problem | Easy | 10 | Solution |
Introduction | Write a function | Problem | Medium | 10 | Solution |
Introduction | Print Function | Problem | Easy | 20 | Solution |
Basic Data Types | List Comprehensions | Problem | Easy | 10 | Solution |
Basic Data Types | Find the Runner-Up Score! | Problem | Easy | 10 | Solution |
Basic Data Types | Nested Lists | Problem | Easy | 10 | Solution |
Basic Data Types | Finding the percentage | Problem | Easy | 10 | Solution |
Basic Data Types | Lists | Problem | Easy | 10 | Solution |
Basic Data Types | Tuples | Problem | Easy | 10 | Solution |
Strings | sWAP cASE | Problem | Easy | 10 | Solution |
Strings | String Split and Join | Problem | Easy | 10 | Solution |
Strings | What's Your Name? | Problem | Easy | 10 | Solution |
Strings | Mutations | Problem | Easy | 10 | Solution |
Strings | Find a string | Problem | Easy | 10 | Solution |
Strings | String Validators | Problem | Easy | 10 | Solution |
Strings | Text Alignment | Problem | Easy | 10 | Solution |
Strings | Text Wrap | Problem | Easy | 10 | Solution |
Strings | Designer Door Mat | Problem | Easy | 10 | Solution |
Strings | String Formatting | Problem | Easy | 10 | Solution |
Strings | Alphabet Rangoli | Problem | Easy | 20 | Solution |
Strings | Capitalize! | Problem | Easy | 20 | Solution |
Strings | The Minion Game | Problem | Medium | 40 | Solution |
Strings | Merge the Tools! | Problem | Medium | 40 | Solution |
Sets | Introduction to Sets | Problem | Easy | 10 | Solution |
Sets | No Idea! | Problem | Medium | 50 | Solution |
Sets | Symmetric Difference | Problem | Easy | 10 | Solution |
Sets | Set add() | Problem | Easy | 10 | Solution |
Sets | Set discard() remove() pop() | Problem | Easy | 10 | Solution |
Sets | Set union() Operation | Problem | Easy | 10 | Solution |
Sets | Set intersection() Operation | Problem | Easy | 10 | Solution |
Sets | Set difference() Operation | Problem | Easy | 10 | Solution |
Sets | Set symmetric_difference() Operation | Problem | Easy | 10 | Solution |
Sets | Set Mutations | Problem | Easy | 10 | Solution |
Sets | The Captain's Room | Problem | Easy | 10 | Solution |
Sets | Check Subset | Problem | Easy | 10 | Solution |
Sets | Check Strict Superset | Problem | Easy | 10 | Solution |
Math | Polar Coordinates | Problem | Easy | 10 | Solution |
Math | Find Angle MBC | Problem | Medium | 10 | Solution |
Math | Triangle Quest 2 | Problem | Medium | 20 | Solution |
Math | Mod Divmod | Problem | Easy | 10 | Solution |
Math | Power - Mod Power | Problem | Easy | 10 | Solution |
Math | Integers Come In All Sizes | Problem | Easy | 10 | Solution |
Math | Triangle Quest | Problem | Medium | 20 | Solution |
Itertools | itertools.product() | Problem | Easy | 10 | Solution |
Itertools | itertools.permutations() | Problem | Easy | 10 | Solution |
Itertools | itertools.combinations() | Problem | Easy | 10 | Solution |
Itertools | itertools.combinations_with_replacement() | Problem | Easy | 10 | Solution |
Itertools | Compress the String! | Problem | Medium | 20 | Solution |
Itertools | Iterables and Iterators | Problem | Medium | 40 | Solution |
Itertools | Maximize It! | Problem | Hard | 50 | Solution |
Collections | collections.Counter() | Problem | Easy | 10 | Solution |
Collections | defaultdict Tutorial | Problem | Easy | 20 | Solution |
Collections | collections.namedtuple() | Problem | Easy | 20 | Solution |
Collections | collections.OrderedDict() | Problem | Easy | 20 | Solution |
Collections | collections.deque() | Problem | Easy | 20 | Solution |
Collections | Company Logo | Problem | Medium | 40 | Solution |
Collections | Word Order | Problem | Medium | 50 | Solution |
Collections | Piling Up! | Problem | Medium | 50 | Solution |
Date and Time | Calendar Module | Problem | Easy | 10 | Solution |
Date and Time | Time Delta | Problem | Medium | 30 | Solution |
Errors and Exceptions | Exceptions | Problem | Easy | 10 | Solution |
Errors and Exceptions | Incorrect Regex | Problem | Easy | 20 | Solution |
Classes | Classes - Dealing with Complex Numbers | Problem | Medium | 20 | Solution |
Classes | Class 2 - Find the Torsional Angle | Problem | Easy | 20 | Solution |
Subdomain | Challenge | Problem | Difficulty | Score | Solution |
---|---|---|---|---|---|
Introduction | Matching Specific String | Problem | Easy | 5 | Solution |
Introduction | Matching Anything But a Newline | Problem | Easy | 5 | Solution |
Introduction | Matching Digits & Non-Digit Characters | Problem | Easy | 5 | Solution |
Introduction | Matching Whitespace & Non-Whitespace Character | Problem | Easy | 5 | Solution |
Introduction | Matching Word & Non-Word Character | Problem | Easy | 5 | Solution |
Introduction | Matching Start & End | Problem | Easy | 5 | Solution |
Character Class | Matching Specific Characters | Problem | Easy | 10 | Solution |
Character Class | Excluding Specific Characters | Problem | Easy | 10 | Solution |
Character Class | Matching Character Ranges | Problem | Easy | 10 | Solution |
Repetitions | Matching {x} Repetitions | Problem | Easy | 20 | Solution |
Repetitions | Matching {x, y} Repetitions | Problem | Easy | 20 | Solution |
Repetitions | Matching Zero Or More Repetitions | Problem | Easy | 20 | Solution |
Repetitions | Matching One Or More Repetitions | Problem | Easy | 20 | Solution |
Repetitions | Matching Ending Items | Problem | Easy | 20 | Solution |
Grouping and Capturing | Matching Word Boundaries | Problem | Easy | 20 | Solution |
Grouping and Capturing | Capturing & Non-Capturing Groups | Problem | Easy | 20 | Solution |
Grouping and Capturing | Alternative Matching | Problem | Easy | 20 | Solution |
Backreferences | Matching Same Text Again & Again | Problem | Easy | 20 | Solution |
Backreferences | Backreferences To Failed Groups | Problem | Easy | 20 | Solution |
Backreferences | Branch Reset Groups | Problem | Easy | 20 | Solution |
Backreferences | Forward References | Problem | Easy | 20 | Solution |
Assertions | Positive Lookahead | Problem | Easy | 20 | Solution |
Assertions | Negative Lookahead | Problem | Easy | 20 | Solution |
Assertions | Positive Lookbehind | Problem | Easy | 20 | Solution |
Assertions | Negative Lookbehind | Problem | Easy | 20 | Solution |
Subdomain | Challenge | Problem | Difficulty | Score | Solution |
---|---|---|---|---|---|
Functions | Security Functions | Problem | Easy | 5 | Solution |
Functions | Security Functions II | Problem | Easy | 5 | Solution |
Functions | Security Bijective Functions | Problem | Easy | 10 | Solution |
Functions | Security Function Inverses | Problem | Easy | 10 | Solution |
Functions | Security Permutations | Problem | Easy | 10 | Solution |
Functions | Security Involution | Problem | Easy | 10 | Solution |
Terminology and Concepts | Security - Message Space and Ciphertext Space | Problem | Easy | 10 | Solution |
Terminology and Concepts | Security Key Spaces | Problem | Easy | 10 | Solution |
Terminology and Concepts | Security Encryption Scheme | Problem | Easy | 10 | Solution |
Cryptography | PRNG Sequence Guessing | Problem | Medium | 50 | Solution |
Cryptography | Keyword Transposition Cipher | Problem | Easy | 50 | Solution |
Cryptography | Basic Cryptanalysis | Problem | Hard | 50 | Solution |
Subdomain | Challenge | Problem | Difficulty | Score | Solution |
---|---|---|---|---|---|
Basic Select | Reversing the Select Query I | Problem | Easy | 10 | Solution |
Basic Select | Revising the Select Query II | Problem | Easy | 10 | Solution |
Basic Select | Select All | Problem | Easy | 10 | Solution |
Basic Select | Select By ID | Problem | Easy | 10 | Solution |
Basic Select | Japanese Cities' Attributes | Problem | Easy | 10 | Solution |
Basic Select | Japanese Cities' Names | Problem | Easy | 10 | Solution |
Basic Select | Weather Observation Station 1 | Problem | Easy | 15 | Solution |
Basic Select | Weather Observation Station 3 | Problem | Easy | 10 | Solution |
Basic Select | Weather Observation Station 4 | Problem | Easy | 10 | Solution |
Basic Select | Weather Observation Station 5 | Problem | Easy | 30 | Solution |
Basic Select | Weather Observation Station 6 | Problem | Easy | 10 | Solution |
Basic Select | Weather Observation Station 7 | Problem | Easy | 10 | Solution |
Basic Select | Weather Observation Station 8 | Problem | Easy | 15 | Solution |
Basic Select | Weather Observation Station 9 | Problem | Easy | 10 | Solution |
Basic Select | Weather Observation Station 10 | Problem | Easy | 10 | Solution |
Basic Select | Weather Observation Station 11 | Problem | Easy | 15 | Solution |
Basic Select | Weather Observation Station 12 | Problem | Easy | 15 | Solution |
Basic Select | Higher Than 75 Marks | Problem | Easy | 15 | Solution |
Basic Select | Employee Names | Problem | Easy | 10 | Solution |
Basic Select | Employee Salaries | Problem | Easy | 10 | Solution |
Advanced Select | Type of Triangle | Problem | Easy | 20 | Solution |
Advanced Select | The PADS | Problem | Medium | 30 | Solution |
Advanced Select | Occupations | Problem | Medium | 30 | Solution |
Advanced Select | Binary Tree Nodes | Problem | Medium | 30 | Solution |
Advanced Select | New Companies | Problem | Medium | 30 | Solution |
Aggregation | Revising Aggregations - The Count Function | Problem | Easy | 10 | Solution |
Aggregation | Revising Aggregations - The Sum Function | Problem | Easy | 10 | Solution |
Aggregation | Revising Aggregations - Averages | Problem | Easy | 10 | Solution |
Aggregation | Average Population | Problem | Easy | 10 | Solution |
Aggregation | Japan Population | Problem | Easy | 10 | Solution |
Aggregation | Population Density Difference | Problem | Easy | 10 | Solution |
Aggregation | The Blunder | Problem | Easy | 15 | Solution |
Aggregation | Top Earners | Problem | Easy | 20 | Solution |
Aggregation | Weather Observation Station 2 | Problem | Easy | 15 | Solution |
Aggregation | Weather Observation Station 13 | Problem | Easy | 10 | Solution |
Aggregation | Weather Observation Station 14 | Problem | Easy | 10 | Solution |
Aggregation | Weather Observation Station 15 | Problem | Easy | 15 | Solution |
Aggregation | Weather Observation Station 16 | Problem | Easy | 10 | Solution |
Aggregation | Weather Observation Station 17 | Problem | Easy | 15 | Solution |
Aggregation | Weather Observation Station 18 | Problem | Medium | 25 | Solution |
Aggregation | Weather Observation Station 19 | Problem | Medium | 30 | Solution |
Aggregation | Weather Observation Station 20 | Problem | Medium | 40 | Solution |
Day | Challenge | Problem | Difficulty | Score | Solution |
---|---|---|---|---|---|
0 | Hello, World! | Problem | Easy | 10 | Solution |
0 | Data Types | Problem | Easy | 10 | Solution |
1 | Arithmetic Operators | Problem | Easy | 10 | Solution |
1 | Functions | Problem | Easy | 10 | Solution |
1 | Let and Const | Problem | Easy | 10 | Solution |
2 | Conditional Statements - If-Else | Problem | Easy | 10 | Solution |
2 | Conditional Statements - Switch | Problem | Easy | 10 | Solution |
2 | Loops | Problem | Easy | 10 | Solution |
3 | Arrays | Problem | Easy | 15 | Solution |
3 | Try, Catch, and Finally | Problem | Easy | 15 | Solution |
3 | Throw | Problem | Easy | 15 | Solution |
4 | Create a Rectangle Object | Problem | Easy | 15 | Solution |
4 | Count Objects | Problem | Easy | 15 | Solution |
4 | Classes | Problem | Easy | 15 | Solution |
5 | Inheritance | Problem | Easy | 15 | Solution |
5 | Template Literals | Problem | Easy | 15 | Solution |
5 | Arrow Functions | Problem | Easy | 15 | Solution |
6 | Bitwise Operators | Problem | Easy | 15 | Solution |
6 | JavaScript Dates | Problem | Easy | 15 | Solution |
7 | Regular Expressions I | Problem | Easy | 15 | Solution |
7 | Regular Expressions II | Problem | Easy | 15 | Solution |
7 | Regular Expressions III | Problem | Easy | 15 | Solution |
8 | Create a Button | Problem | Easy | 20 | Solution |
8 | Buttons Container | Problem | Easy | 25 | Solution |
9 | Binary Calculator | Problem | Medium | 30 | Solution |
Day | Challenge | Problem | Difficulty | Score | Solution |
---|---|---|---|---|---|
0 | Mean, Median, and Mode | Problem | Easy | 30 | Solution |
0 | Weighted Mean | Problem | Easy | 30 | Solution |
1 | Quartiles | Problem | Easy | 30 | Solution |
1 | Interquartile Range | Problem | Easy | 30 | Solution |
1 | Standard Deviation | Problem | Easy | 30 | Solution |
2 | Basic Probability | Problem | Easy | 10 | Solution |
2 | More Dice | Problem | Easy | 10 | Solution |
2 | Compound Event Probability | Problem | Easy | 10 | Solution |
3 | Conditional Probability | Problem | Easy | 10 | Solution |
3 | Cards of the Same Suit | Problem | Easy | 10 | Solution |
3 | Drawing Marbles | Problem | Easy | 10 | Solution |
4 | Binomial Distribution I | Problem | Easy | 30 | Solution |
4 | Binomial Distribution II | Problem | Easy | 30 | Solution |
4 | Geometric Distribution I | Problem | Easy | 30 | Solution |
4 | Geometric Distribution II | Problem | Easy | 30 | Solution |
5 | Poisson Distribution I | Problem | Easy | 30 | Solution |
5 | Poisson Distribution II | Problem | Easy | 30 | Solution |
5 | Normal Distribution I | Problem | Easy | 30 | Solution |
5 | Normal Distribution II | Problem | Easy | 30 | Solution |
6 | The Central Limit Theorem I | Problem | Easy | 30 | Solution |
6 | The Central Limit Theorem II | Problem | Easy | 30 | Solution |
6 | The Central Limit Theorem III | Problem | Easy | 30 | Solution |
7 | Pearson Correlation Coefficient I | Problem | Easy | 30 | Solution |
7 | Spearman's Rank Correlation Coefficient | Problem | Easy | 30 | Solution |
8 | Least Square Regression Line | Problem | Easy | 30 | Solution |
8 | Pearson Correlation Coefficient II | Problem | Easy | 30 | Solution |
9 | Multiple Linear Regression | Problem | Medium | 30 | Solution |
Day | Challenge | Problem | Difficulty | Score | Python | C# |
---|---|---|---|---|---|---|
0 | Hello, World | Problem | Easy | 30 | Solution | Solution |
1 | Data Types | Problem | Easy | 30 | Solution | Solution |
2 | Operators | Problem | Easy | 30 | Solution | Solution |
3 | Intro to Conditional Statements | Problem | Easy | 30 | Solution | Solution |
4 | Class vs. Instance | Problem | Easy | 30 | Solution | Solution |
5 | Loops | Problem | Easy | 30 | Solution | Solution |
6 | Let's Review | Problem | Easy | 30 | Solution | Solution |
7 | Arrays | Problem | Easy | 30 | Solution | Solution |
8 | Dictionaries and Maps | Problem | Easy | 30 | Solution | Solution |
9 | Recursion 3 | Problem | Easy | 30 | Solution | Solution |
10 | Binary Numbers | Problem | Easy | 30 | Solution | Solution |
11 | 2D Arrays | Problem | Easy | 30 | Solution | Solution |
12 | Inheritance | Problem | Easy | 30 | Solution | Solution |
13 | Abstract Classes | Problem | Easy | 30 | Solution | Solution |
14 | Scope | Problem | Easy | 30 | Solution | Solution |
15 | Linked List | Problem | Easy | 30 | Solution | Solution |
16 | Exceptions - String to Integer | Problem | Easy | 30 | Solution | Solution |
17 | More Exceptions | Problem | Easy | 30 | Solution | Solution |
18 | Queues and Stack | Problem | Easy | 30 | Solution | Solution |
19 | Interfaces | Problem | Easy | 30 | Solution | Solution |
20 | Sorting | Problem | Easy | 30 | Solution | Solution |
21 | Generics | Problem | Easy | 30 | Solution | Solution |
22 | Binary Search Trees | Problem | Easy | 30 | Solution | Solution |
23 | BST Level-Order Traversal | Problem | Easy | 30 | Solution | Solution |
24 | More Linked Lists | Problem | Easy | 30 | Solution | Solution |
25 | Running Time and Complexity | Problem | Medium | 30 | Solution | Solution |
26 | Nested Logic | Problem | Easy | 30 | Solution | Solution |
27 | Testing | Problem | Easy | 30 | Solution | Solution |
28 | RegEx, Patterns, and Intro to Databases | Problem | Medium | 30 | Solution | Solution |
29 | Bitwise AND | Problem | Medium | 30 | Solution | Solution |
Domain | Challenge | Problem | Difficulty | Score | Solution |
---|---|---|---|---|---|
Warm-up Challenges | Sock Merchant | Problem | Easy | 10 | Solution |
Warm-up Challenges | Counting Valleys | Problem | Easy | 15 | Solution |
Warm-up Challenges | Jumping on the Clouds | Problem | Easy | 20 | Solution |
Warm-up Challenges | Repeated String | Problem | Easy | 20 | Solution |
Arrays | 2D Arrays - DS | Problem | Easy | 15 | Solution |
Arrays | Arrays - Left Rotation | Problem | Easy | 20 | Solution |
Arrays | New Year Chaos | Problem | Medium | 40 | Solution |
Arrays | Minimum Swaps 2 | Problem | Medium | 40 | Solution |
Arrays | Array Manipulation | Problem | Hard | 60 | Solution |
Dictionaries and Hashmaps | Hash Tables - Ransom Note | Problem | Easy | 25 | Solution |
Dictionaries and Hashmaps | Two Strings | Problem | Easy | 25 | Solution |
Dictionaries and Hashmaps | Sherlock and Anagrams | Problem | Medium | 50 | Solution |
Dictionaries and Hashmaps | Count Triplets | Problem | Medium | 35 | Solution |
Dictionaries and Hashmaps | Frequency Queries | Problem | Medium | 40 | Solution |
Sorting | Sorting - Bubble Sort | Problem | Easy | 30 | Solution |
Sorting | Mark and Toys | Problem | Easy | 35 | Solution |
Sorting | Sorting - Comparator | Problem | Medium | 35 | Solution |
Sorting | Fraudulent Activity Notifications | Problem | Medium | 40 | Solution |
Sorting | Merge Sort - Counting Inversions | Problem | Hard | 45 | Solution |
String Manipulation | Strings - Making Anagrams | Problem | Easy | 25 | Solution |
String Manipulation | Alternating Characters | Problem | Easy | 20 | Solution |
String Manipulation | Sherlock and the Valid String | Problem | Medium | 35 | Solution |
String Manipulation | Special String Again | Problem | Medium | 40 | Solution |
String Manipulation | Common Child | Problem | Hard | 60 | Solution |
Greedy Algorithm | Minimum Absolute Difference in an Array | Problem | Easy | 15 | Solution |
Greedy Algorithm | Luck Balance | Problem | Easy | 20 | Solution |
Greedy Algorithm | Greedy Florist | Problem | Medium | 35 | Solution |
Greedy Algorithm | Max Min | Problem | Medium | 35 | Solution |
Greedy Algorithm | Reverse Shuffle Merge | Problem | Advanced | 50 | Solution |
Skill | Test | Difficulty | Certificate |
---|---|---|---|
Python | Test | Basic | Certificate |
Java | Test | Basic | Certificate |
JavaScript | Test | Basic | Certificate |
React | Test | Basic | Certificate |
Problem Solving | Test | Basic | Certificate |