CLICK HERE! to launch HTML/CSS Advanced website link
What is HTML
HTML stands for HyperText Markup Language. It's the standard markup language used to create web pages. HTML provides the structure for the content that appears on web browsers. Think of it like the "skeleton" of a web page, providing a place for other technologies like CSS (styling) and JavaScript (interactivity) to attach to.How to create an HTML page from a wireframe
Look at your wireframe and identify the elements you'll need (headers, paragraphs, links, images, etc.).Create HTML Skeleton: Start with the basic HTML structure (<!DOCTYPE html>, <html>, <head>, <body>).
Add Semantic Elements: Use HTML tags that correspond to the elements you identified in your wireframe. Add them within the <body> of your HTML document.
Annotate with Comments: If your WireFrame is complex, you might add HTML comments (<!-- like this -->) to describe what each section is for.
Fill in Attributes: Where needed, add attributes to your elements. For example, you'll need href attributes for links and src attributes for images.
Review and Test: Double-check your code against your WireFrame. Make sure each part of the WireFrame is represented in your HTML structure.
What is a markup language
A markup language is a system for annotating a document in a way that is syntactically distinguishable from the text. In simpler terms, it's a way to describe and structure your content. For example, in HTML, you use tags like <p> for paragraphs and <h1> for a level-one header.What is the DOM
DOM stands for Document Object Model. It's a programming interface that allows you to interact with HTML (and XML) documents programmatically. When a web page is loaded, the browser creates the DOM of the page, which is an object-oriented representation of the web page's structure.What is an element / tag
An element or tag in HTML defines the structure and content in an HTML document. Elements are written with a start tag, some content, and an end tag. For example, a paragraph is created using the <p> (start tag), some text, and </p> (end tag).What is an attribute
Attributes provide additional information about an element. They're placed within the opening tag. For example, in <img src="image.jpg" alt="An image"><img src="image.jpg" alt="An image">, src and alt are attributes that specify the image source and alternative text, respectively.What the purpose of each HTML tag
Each tag serves a specific purpose in creating and laying out content.<html>: Root element that contains all other HTML elements.
<head>: Contains meta information, links to CSS, JS files, etc.
<title>: Sets the title of the web page.
<body>: Contains the content of the web page.
<h1>, <h2>, ... <h6>: Headings to structure content.
<p>: Paragraph.
<a>: Anchor (links).
<img>: Embed images.
<ul>, <ol>, <li>: Lists.
<table>, <tr>, <td>: Table and its rows and data cells.
... and many more.
CSS stands for Cascading Style Sheets. It is a stylesheet language used to describe how HTML elements should look on a webpage.
HTML structures the content, CSS styles it. You can change font sizes, colors, add margins, and much more using CSS. It is important because CSS makes web pages visually appealing as well as
separating content (HTML) from styling (CSS), making it easier to maintain.
- Inline Style: Directly within the HTML element using the 'style' attribute.
<p style="color: red;">This is red text.</p>
- Internal Style Sheet: Within the HTML file but inside the <head> section using <style> tags.
<style>
p {
color: red;
}
</style>
- External Style Sheet: In a separate .css file, linked to the HTML file.
/* styles.css */
p {
color: red;
}
<!-- index.html -->
<link rel="stylesheet" href="styles.css">
- Best Practices
Use external stylesheets for larger projects for better organization and reusability.
In CSS, a class is a way to select multiple elements that share the same styling. Unlike IDs, which are unique, classes can be reused. This allows you to apply the same style to multiple elements without repeating code.
.my-class {
font-size: 20px;
color: blue;
}
A selector in CSS is used to target HTML elements and apply styles to them. They are the building blocks of CSS, allowing you to target what you want to style.
Types of selectors include:
- Type selectors: Target by element type (p, h1)
- Class selectors: Target by class (.my-class)
- ID selectors: Target by ID (#my-id)
The specificity of a CSS rule is determined by a set of rules usually calculated in a (0,0,0,0) format:
- !Important flagged styles.
([#]-0-0-0-0) - Inline styles: Highest specificity.
([#]-0-0-0) - IDs: More specific than any class.
([#]-0-0) - Classes, attributes, and pseudo-classes: Next level down.
(0-[#]-0) - Type selectors and pseudo-elements: Lowest level.
(0-0-[#])
EXAMPLES:
- ' .my-class p ': (0,1,1) - 0 IDs, 1 Class, 1 Element
- ' #my-id .my-class ': (1,1,0) - 1 ID, 1 Class, 0 Elements
These properties help in arranging elements properly on a page. These properties control layout:
- Margin: Outside the border.
- Border: Surrounds the padding and content.
- Padding: Inside the border, outside the content.
- Content: The actual content.
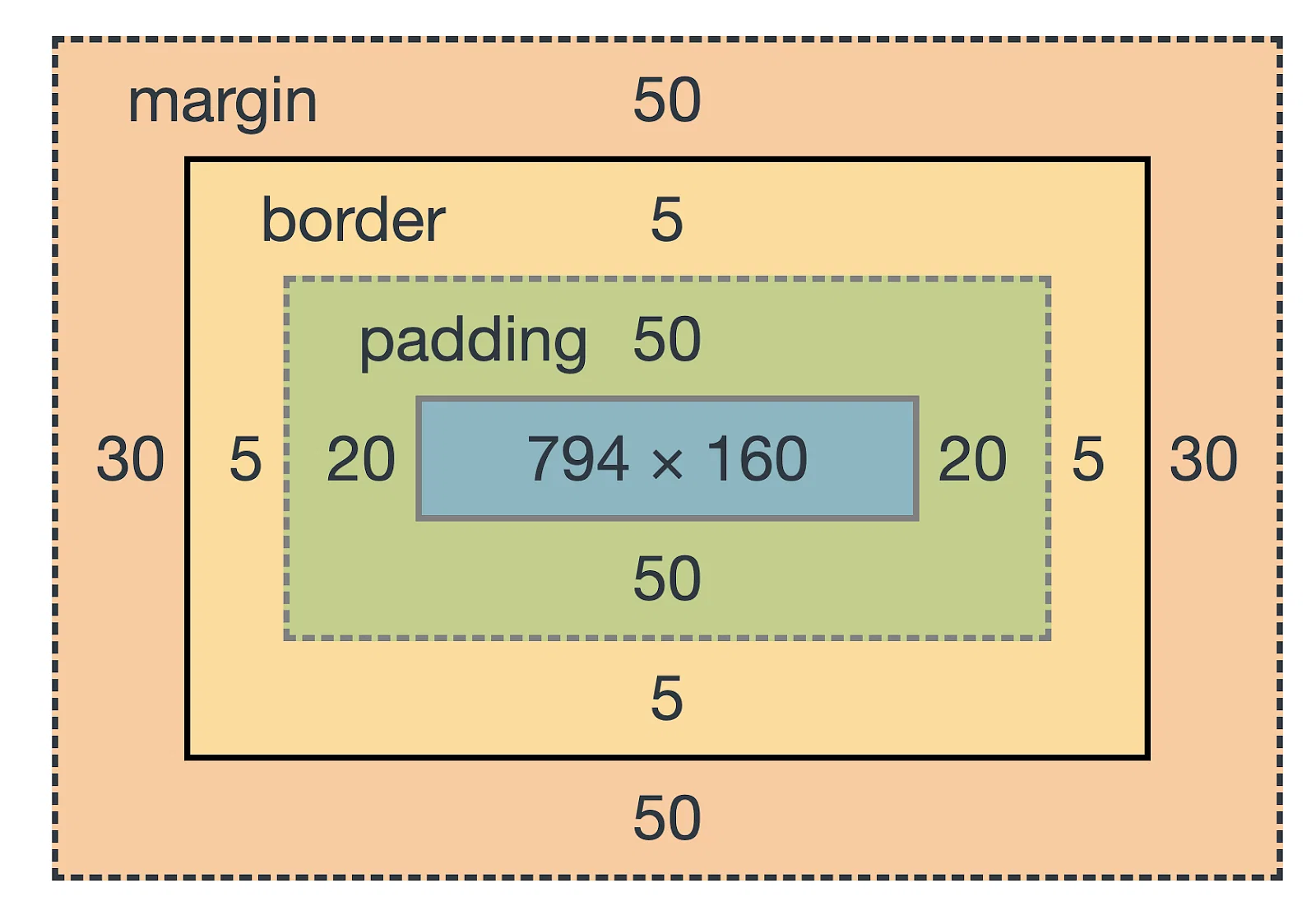
- DNS Lookup: Translates URL to IP address.
- The browser converts the human-friendly URL into an IP address.
- HTTP Request: Browser requests data.
- The browser asks the server at that IP address for the webpage content.
- Server Response: Sends back files.
- The server sends back the necessary files like HTML, CSS, and JS.
- Browser Render: Parses HTML and CSS, runs JS, and renders the page.
- Finally, your browser combines these files to display the webpage you see.