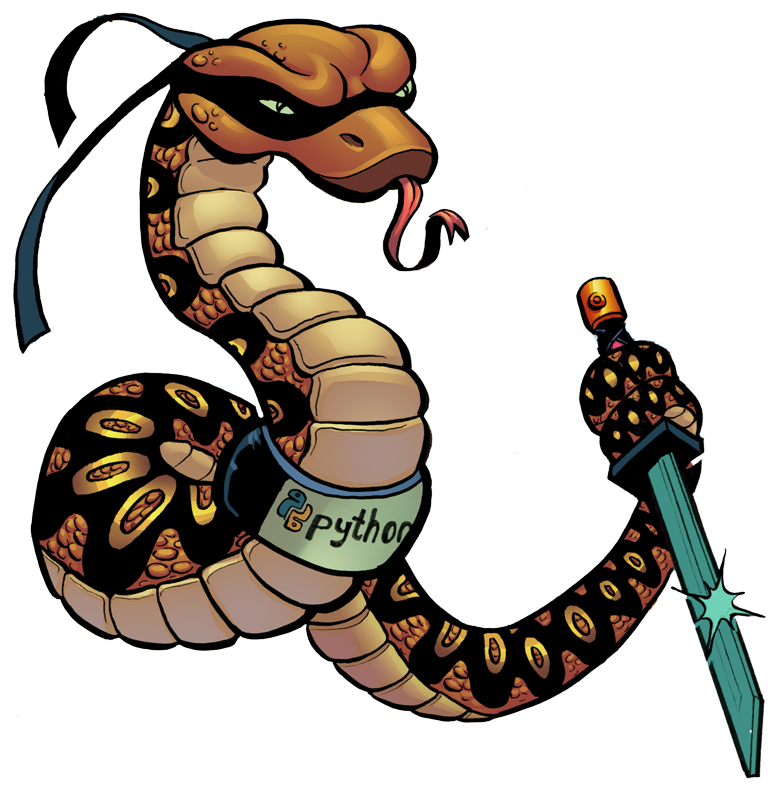
The repository is a comprehensive guide tailored to enhance Python skills. It caters to both beginners and experienced developers, offering in-depth tutorials, practical examples, and hands-on exercises covering a wide range of Python topics. From fundamental concepts to advanced techniques, each section aims to equip learners with the knowledge and skills to navigate Python proficiently. The repository provides a structured and easily understandable approach, facilitating quick progress for learners. Additionally, it offers insights into techniques applicable to other programming languages and fosters community engagement for skill improvement. Whether you're starting out or seeking to refine your Python expertise, this repository serves as an invaluable resource.
1. Python Basics 📚
- Syntax
- Comments
- Variables
- Constants
- Data_Types
- Type_Hints
- Shortcut_Format
- Integers
- Floats
- Operators
- Strings
- Type_Conversion
- Simple_Adder_Project
- Booleans
- Lists
- Tuples
- Sets
- Frozensets
- Dictionaries
- None
- Mad_Libs_Project
- Truthy_and_Falsy
- Comparing_Floats
- Scopes
- Global
- Nonlocal
- Doc_Strings
- F-Strings
- Assertions
- Unpacking
- __ VS __ == Is
2. Control Flow 🔄
3. Functions 🎯
5. Modules 📦
6. Lists 📋
7. OOP 🏗️
8. Built in Functions 🛠️
9. Dataclasses 📇
10. AsyncIO ⚙️
11. Advanced 🚀
12. AsyncIO ⚙️
- Introduction
- Tasks
- Gather
- Website Status
13. Multithreading 🔄
- Threads
- Locks
- Daemon-Threads
- Semaphores
- With Lock Semaphore
- Race Conditions
14. Multiprocessing 🔄
- Processes
- Pools Map
- Pools Starmap
- Pools Multiple Functions
- Data Sharing Issue
- Pipes
- Queues
- Lock & Semaphores
15. Unit-Testing ✔️
- Fixtures
- Conftest
- Marks
- Parametrize
- Testing Errors
16. File-Management 📁
- File Handling
- Reading Files
- Writing Creating
- Deleting Files
- JSON
- Handling JSON
- Caching JSON
- Glob
- Pickling
- YAML
- TOML
17. Design Patterns 🎨
- Creational Patterns
- Structural Patterns
- Behavioral Patterns
18. GUI
- Text
- Text
- Text
- Text
19. Tips & Tricks 🎨
- F-Strings
- Tuples & Type Hinting
- Flattening Lists
- Elipses
- Linting
- #NOQA
- Concatenating Strings
- Backslashes
- Underscores
- List vs Arrays
- IIFE in Python