Adds emojis and infers commit context for people who use conventional commits
- install it as git hooks:
prepare-commit-msg
and commit-msg
- follow git conventional commits: https://www.conventionalcommits.org/en/v1.0.0-beta.2/
- ???
- PROFIT
Open GIF spoiler
demo on streamable: https://streamable.com/n0u5p
webm: https://github.com/ColCh/git-commit-msg/blob/master/demo/demo.webm
This hook currently does that:
- Prepend emoji before git commit type. Predefined types are here:
|
const commitTypeToEmoji = { |
|
chore: '😒', |
|
docs: '📝', |
|
feat: '⭐', |
|
fix: '🛠', |
|
refactor: '♻', |
|
style: '💄', |
|
test: '🔍', |
|
type: '🏷', |
|
perf: '⚡', |
|
ci: '🤖', |
|
}; |
- Mark words with predefined emoji defined here:
|
const markWordsWithEmoji = { |
|
update: '⬆ ', |
|
add: '➕', |
|
remove: '➖', |
|
change: '🔁', |
|
rename: '🔁', |
|
package: '📦', |
|
deploy: '📦', |
|
fix: '🛠', |
|
webpack: ' 🎁', |
|
increase: '📈 ', |
|
decrease: ' 📉', |
|
copy: ' 📋', |
|
config: '⚙️', |
|
configure: '⚙️', |
|
optimize: ' 🚀', |
|
typo: ' 📝', |
|
}; |
. Example:
|
exports[`main test for git-commit-msg mark words with emoji per-word tests given word "add" should match snapshot 1`] = `"some msg ➕ add some msg"`; |
|
|
|
exports[`main test for git-commit-msg mark words with emoji per-word tests given word "change" should match snapshot 1`] = `"some msg 🔁 change some msg"`; |
|
|
|
exports[`main test for git-commit-msg mark words with emoji per-word tests given word "config" should match snapshot 1`] = `"some msg ⚙️ config some msg"`; |
|
|
|
exports[`main test for git-commit-msg mark words with emoji per-word tests given word "configure" should match snapshot 1`] = `"some msg ⚙️ configure some msg"`; |
|
|
|
exports[`main test for git-commit-msg mark words with emoji per-word tests given word "copy" should match snapshot 1`] = `"some msg 📋 copy some msg"`; |
|
|
|
exports[`main test for git-commit-msg mark words with emoji per-word tests given word "decrease" should match snapshot 1`] = `"some msg 📉 decrease some msg"`; |
|
|
|
exports[`main test for git-commit-msg mark words with emoji per-word tests given word "deploy" should match snapshot 1`] = `"some msg 📦 deploy some msg"`; |
|
|
|
exports[`main test for git-commit-msg mark words with emoji per-word tests given word "fix" should match snapshot 1`] = `"some msg 🛠 fix some msg"`; |
|
|
|
exports[`main test for git-commit-msg mark words with emoji per-word tests given word "increase" should match snapshot 1`] = `"some msg 📈 increase some msg"`; |
|
|
|
exports[`main test for git-commit-msg mark words with emoji per-word tests given word "optimize" should match snapshot 1`] = `"some msg 🚀 optimize some msg"`; |
|
|
|
exports[`main test for git-commit-msg mark words with emoji per-word tests given word "package" should match snapshot 1`] = `"some msg 📦 package some msg"`; |
|
|
|
exports[`main test for git-commit-msg mark words with emoji per-word tests given word "remove" should match snapshot 1`] = `"some msg ➖ remove some msg"`; |
|
|
|
exports[`main test for git-commit-msg mark words with emoji per-word tests given word "rename" should match snapshot 1`] = `"some msg 🔁 rename some msg"`; |
|
|
|
exports[`main test for git-commit-msg mark words with emoji per-word tests given word "typo" should match snapshot 1`] = `"some msg 📝 typo some msg"`; |
|
|
|
exports[`main test for git-commit-msg mark words with emoji per-word tests given word "update" should match snapshot 1`] = `"some msg ⬆ update some msg"`; |
|
|
|
exports[`main test for git-commit-msg mark words with emoji per-word tests given word "webpack" should match snapshot 1`] = `"some msg 🎁 webpack some msg"`; |
- Try to infer git commit context. Example:
|
# Found 3 contexts |
|
# * modified-file |
|
# * deleted-file |
|
# * new-file |
yarn global add @colch/git-commit-msg
add git-commit-msg
into prepare-commit-msg
and commit-msg
hooks:
git-commit-msg $1
to appear like that:
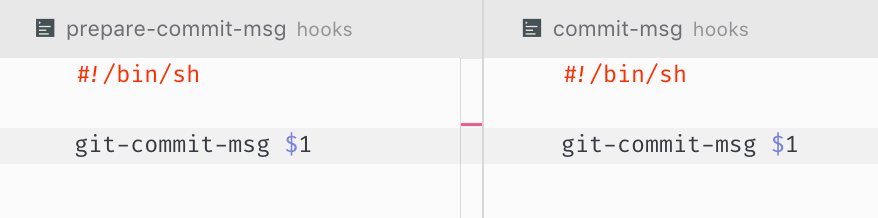
pass it like env variables