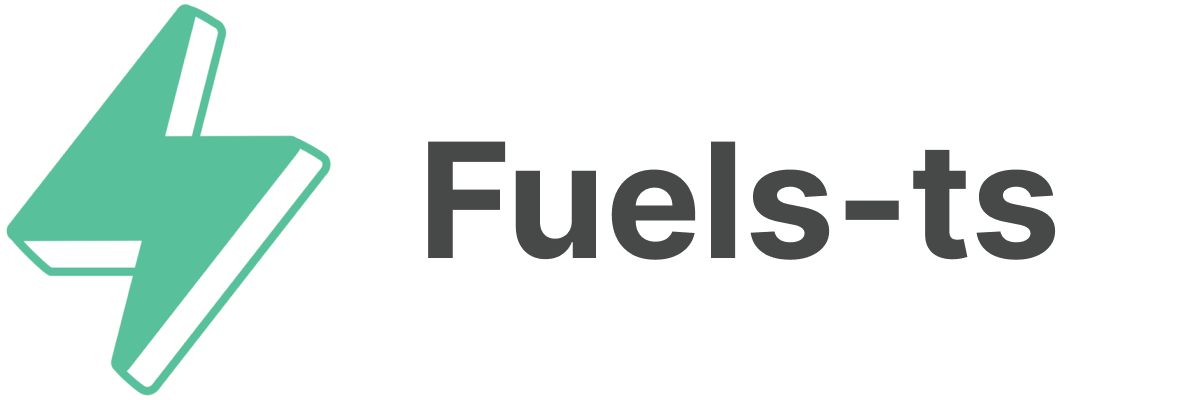
fuels-ts is a library for interacting with Fuel v2.
- Quickstart
- Documentation
- Install
- Import
- Calling Contracts
- Generate Contract Types from ABI
- Deploying Contracts
- Contributing
- License
We recommend to start on Quickstart to speed-up and build your first DApp using Fuel.
Find more information about packages in our Documentation.
Learn more about the Fuel Ecosystem.
- 🌴 Sway the new language. Empowering everyone to build reliable and efficient smart contracts.
- 🧰 Forc the Fuel toolbox. Build, deploy and manage your sway projects.
- ⚙️ Fuel Core the new FuelVM, a blazingly fast blockchain VM.
- 🔗 Fuel Specs the Fuel protocol specifications.
- 🦀 RUST SDK a robust SDK in rust.
- ⚡ Fuel Network the project.
yarn add fuels
npm install fuels --save
import { Wallet } from "fuels";
// Random Wallet
console.log(Wallet.generate());
// Using privateKey Wallet
console.log(new Wallet("0x0000...0000"));
import { Wallet, Contract, BigNumberish, BN } from "fuels";
import abi from "./abi.json";
const wallet = new Wallet("0x..."); // private key with coins
const contractId = "0x...";
const contract = new Contract(contractId, abi, wallet);
// All contract methods are available under functions
// with the correct types
const { transactionId, value } = await contract.functions
.foo<[BigNumberish], BN>("bar")
.call();
console.log(transactionId, value);
yarn add -D fuelchain typechain-target-fuels
yarn exec fuelchain --target=fuels --out-dir=types abi.json
import { Wallet } from "fuels";
import { MyContract__factory } from "./types";
const contractId = "0x...";
const wallet = new Wallet("0x...");
const contract = MyContract__factory.connect(contractId, wallet);
// All contract methods are available under functions
// with the correct types
const { transactionId, value } = await contract.functions.my_fn(1n).call();
console.log(transactionId, value);
import { Provider, Contract } from "fuels";
// Byte code generated using: forc build
import bytecode from "./bytecode.bin";
const factory = new ContractFactory(bytecode, [], wallet);
const contract = await factory.deployContract(factory);
console.log(contract.id);
The primary license for this repo is Apache 2.0
, see LICENSE
.