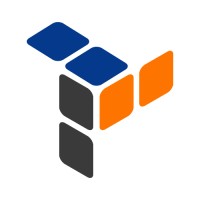
This is an effort by [IncubXperts](https://incubxperts.com) to provide commonly used source code snippets as open source code to community. Feel free to use it in your projects, suggest improvements, report bugs to improve the code for community. Feel free to contribute.
Source code example to generate a thumbnail from an image.
Report Bug
·
Request Feature
·
Request new source code
Table of Contents
Source code example to generate a thumbnail from an image. In our software projects many times, we need to generate image thumbnails. For example, If a user is uploading an image to your web application and displays it on UI. Generally, we show such images in small sizes compared to the actual image size. It's always a better idea to generate a thumbnail to show on UI and when the user clicks on the thumbnail image we should show full-size images only when needed. This saves bandwidth and makes the application load faster.
This sample source code provides an example to create a thumbnail from a full sized image. Typical use in web application The user uploads an image to the application Make the image available as a stream The given code converts the original image to a thumbnail and returns it as a stream. Store the thumbnail image along with the original image for display purposes.
Console app and sample code in C# class.
- [![C#][C Sharp Dotnet 6]][https://dotnet.microsoft.com/en-us/download/dotnet/6.0]
This is an example of how you may give instructions on setting up your project locally. To get a local copy up and running follow these simple example steps.
This is an example of how to list things you need to use the software and how to install them.
- Dotnet 6
- Works on Linux as well as windows.
- Understand the code and use in your project as fit.
static void GenerateThumbnailFromFile(string InputImageFile, string OutputThumbnailImageFile)
{
// Open source file as file stream.
using FileStream inputFileStream = new(InputImageFile, FileMode.Open);
// Call image thumbnail generator to generate thumnail
using (var thumbnailMemStream = ImageThumbnailGenerator.GenerateThumbnail(inputFileStream, 200, 200))
{
var outputFileStream = new FileStream(OutputThumbnailImageFile, FileMode.Create, FileAccess.Write);
thumbnailMemStream.CopyTo(outputFileStream);
outputFileStream.Dispose();
thumbnailMemStream.Dispose();
}
// Close open streams.
inputFileStream.Dispose();
}
Contributions are what make the open source community such an amazing place to learn, inspire, and create. Any contributions you make are greatly appreciated.
If you have a suggestion that would make this better, please fork the repo and create a pull request. You can also simply open an issue with the tag "enhancement". Don't forget to give the project a star! Thanks again!
- Fork the Project
- Create your Feature Branch (
git checkout -b feature/AmazingFeature
) - Commit your Changes (
git commit -m 'Add some AmazingFeature'
) - Push to the Branch (
git push origin feature/AmazingFeature
) - Open a Pull Request
Distributed under the GPL License. See LICENSE.txt
for more information.
IncubXperts - @incubxperts - contact@incubxperts.com
Project Link: https://github.com/IncubXperts/image_thumbnail_csharp