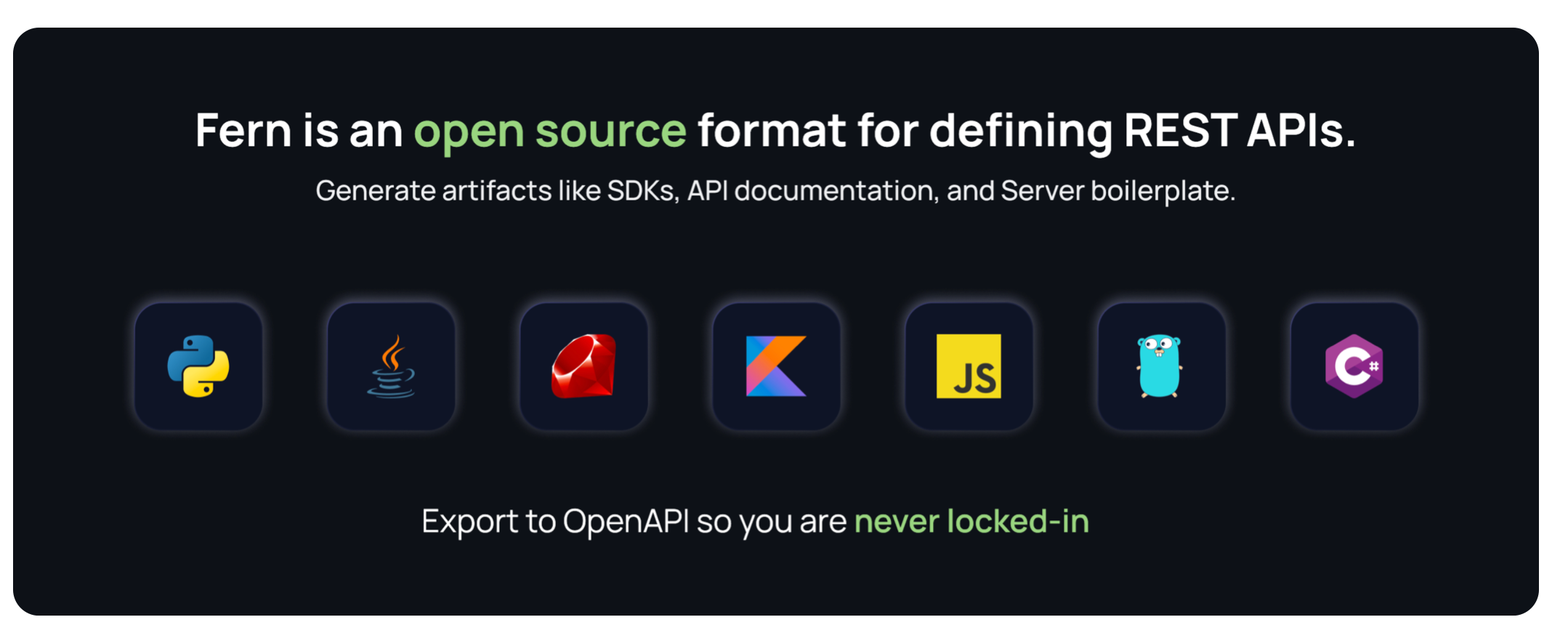
Fern is an open source format for defining REST APIs. You can think of it like a programming language to describe your API: your endpoints, types, errors, and examples.
This repository contains the Fern compiler. The compiler transforms the API description into useful outputs, like:
Client libraries speed up internal developement, and help acquire customers who use your API. Our auto-generated SDKs are idiomatic and feel handwritten.
We automatically generate lots of boilerplate on the server side, like Pydantic models for FastAPI and Jersey interfaces for Spring Boot. We also add compile-time validation that all your endpoints are being served correctly.
Complete with examples of successful and unsuccessful requests!
You can feed the generated OpenAPI into the endless list of tools that support OpenAPI.
OpenAPI is a great tool for documenting APIs, but falls short for code generation use cases.
Read Fern vs. OpenAPI for an in-depth comparison. TL;DR: we differ from OpenAPI in these areas:
- Simplicity
- New features in specification
- Quality of code generation
- Focus on server-side API development
- Change management
- Cloud-based code generation and publishing
Plant Store is full example of an API defined in Fern.
- API Definition
- Generated TypeScript SDK
- Generated Java SDK
- Generated Postman Collection
- Generated OpenAPI spec
npm install -g fern-api
The fern/
directory contains your API definition. This generally lives in your
backend repo, but you can also have an independent repo dedicated to your API (like Raven's).
In the root of your repo, run:
fern init
This will create the following folder structure in your project:
fern/
├─ fern.config.json # root-level configuration
└─ api/ # your API
├─ generators.yml # generators you're using
└─ definition/
├─ api.yml # API-level configuration
└─ imdb.yml # endpoints, types, and errors
Here's what the imdb.yml
starter file looks like:
types:
MovieId: string
Movie:
properties:
id: MovieId
title: string
rating:
type: double
docs: The rating scale is one to five stars
CreateMovieRequest:
properties:
title: string
rating: double
service:
auth: false
base-path: /movies
endpoints:
createMovie:
docs: Add a movie to the database
method: POST
path: /create-movie
request: CreateMovieRequest
response: MovieId
getMovie:
method: GET
path: /{movieId}
path-parameters:
movieId: MovieId
response: Movie
errors:
- MovieDoesNotExistError
errors:
MovieDoesNotExistError:
status-code: 404
type: MovieId
To generate SDKs, you can log in with GitHub from the CLI:
fern login
You can add generators using fern add
. By default, this
will publish your SDK to the Fern npm registry (npm.buildwithfern.com).
fern add fern-typescript-sdk
To generate the TypeScript SDK, run:
fern generate
And voila! You just built and published a TypeScript SDK.
Next step: define your API in Fern. Check out our docs to learn more.
fern generate
runs the compiler. It will validate your API and run the
generators specified in generators.yml
.
If you don't have a default-group
specified in generators.yml
, then you must
specify a group using the --group
option.
You can specify a version using the --version
option. This version string is
used when publishing SDKs to registries (e.g. npm).
fern check
will validate that your API is valid.
fern upgrade
will upgrade your compiler version in fern.config.json
to the
latest version.
fern add
adds a new generator to your generators.yml
. You can view the full
list of supported generators in our docs.
fern init
adds a new API to your repo.
Advanced feature. You can register your API so it can be depended on by other APIs. Read more in our docs.
You can view our documentation here.
Thanks to the folks at Twemoji, an open source project, who created the graphic that we use as our logo.