This is an automated Ethereum agent which signs and calls Ethereum smart contract functions on your behalf via AJAX/XHR GET requests to lambda endpoints. We use it to remove the friction of providing Ether and ERC20 Tokens to our Beta Testers.
You can read the blog post here: https://medium.com/p/fe18b3267c04
A simple example of our Hippocrates ĐApp's frontend showing what this can look like to your beta testers:
You will need the direnv
tool to export environment variables specifically for this project. You can get instructions on how to install it for your machine here: https://direnv.net/
$ npm install
To run the truffle tests:
$ npm run test
Prior to running truffle, the ganache-cli server or the lambda functions you will need to set up your environment variables. Copy the .envrc.example file to .envrc, and update your new .envrc with your unique environment variables:
-
cp .envrc.example .envrc
-
Enter your own wallet's twelve word mnemonic in the .envrc file.
-
Also, we'll leverage Infura's Ethereum Ropsten testnet node, so make sure to set up an account and paste your private key in your .envrc.
-
Use
direnv allow
to export the env vars into your current terminal shell.
The Ganache server acts as a local test Ethereum blockchain and EVM:
$ npm run start
With the Ganache server running you can now compile and migrate (deploy) your contracts to the blockchain. To do this locally run:
npm run migrate
If you want to do this against the Ropsten or Rinkeby blockchains, use:
npm run migrate -- --network=ropsten
or npm run migrate -- --network=rinkeby
If you want your contract to send Ether, you will first need to send Ether to your newly deployed contract. Same goes for any ERC20 token, the contract will need a balance that it can then hand out.
We use truffle console
to send Ether and mint ERC20 token to our deployed BetaFaucet instance. If your BetaFaucet was deployed to the address '0x847e024dfabcacd68cb2c2d120d195ccba4c4484', and your ERC20 token contract was deployed to '0x736768bb1acea596134f39b68bfb877286dd2220' you can mint tokens directly to the deployed BetaFaucet contract.
YTKNToken.at('0x736768bb1acea596134f39b68bfb877286dd2220').then(i => ytknInstance = i)
ytknInstance.mint('0x847e024dfabcacd68cb2c2d120d195ccba4c4484', '100000000000000000000000')
You can easily send your deployed contract Ether via MetaMask, MyCrypto or any other wallet - just as you would send Ether to any other Ethereum address.
If you've deployed your contracts to Ropsten, you can get test Ropsten Ether on any of these faucets:
While your Ganache-CLI server is running (via npm run start
) open another terminal window and install the npm packages in the lambda
dir:
$ cd lambda
$ npm i
$ cd ..
Then use npm run lambda
to compile the lambda functions and start a server at http://localhost:9000
An example of having the contract send you Ether would be to make the following GET request:
http://localhost:9000/betaFaucetSendEther?ethAddress=0x95819594aeb3d6d5abb338b263e944b56dd2f195
After generating your mnemonic and adding it to your .envrc
file, allowing those env vars using direnv allow
, and running the Ganache-CLI server with npm run start
you can find your contract owner's private key at the top of the logs, as the first private key (0):
Awesome! Feel free to open a new Issue or even better, a Pull Request with the fix!
From Amazon's FAQ:
AWS Lambda is a compute service that lets you run code without provisioning or managing servers. AWS Lambda executes your code only when needed and scales automatically, from a few requests per day to thousands per second. You pay only for the compute time you consume - there is no charge when your code is not running.
We use Netlify's Lambda integration. Their 'Functions Free' plan provides you with up to 125,000 requests / month and up to 100 hours of run time / month. You can find more on that here: https://www.netlify.com/docs/functions/
Your contributions are always welcome! Please open a new Issue or Pull Request to contribute. 😄
The Apache License January 2004. Please have a look at the LICENSE for more details.
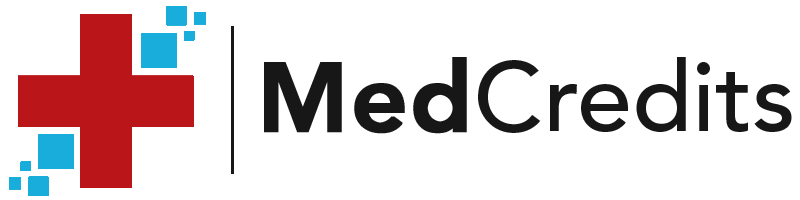