SetStaticsCount |
Loads a value from the code buffer, then sets it as the max statics available for the script (Custom Opcode) |
 |
SetDefaultStatic |
Loads the static index and a value from the code buffer, then defines the value at the index (Custom Opcode) |
 |
SetStaticName |
Loads the static index and a string from the code buffer, then defines the string as the index (Custom Opcode) |
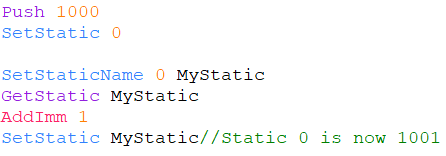 |
SetLocalName |
Loads the local index and a string from the code buffer, then defines the string as the index (Custom Opcode) |
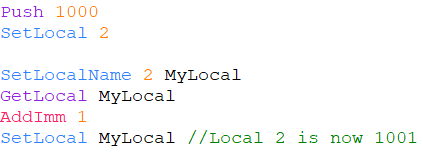 |
SetGlobalName |
Loads the global index and a string from the code buffer, then defines the string as the index (Custom Opcode) |
 |
SetEnum |
Loads the string and a value from the code buffer, then defines the string as the value (Custom Opcode) |
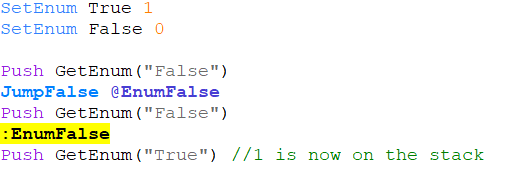 |
Nop |
No Operation / Padding |
 |
Add |
Adds the top two integers on the stack |
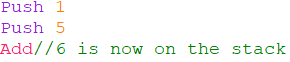 |
Sub |
Subtracts the top two integers on the stack |
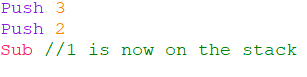 |
Mult |
Multiplies the top two integers on the stack |
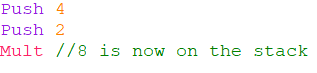 |
Div |
Divides the top two integers on the stack (Checks if the divisor is 0) |
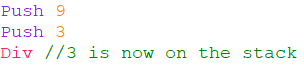 |
Mod |
Performs a modulus on the top two integers on the stack |
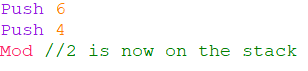 |
Not |
Performs a logical not on the top integer on the stack |
 |
Neg |
Performs a negate on the top integer on the stack |
 |
CmpEq |
Checks if the top two integers on the stack are equal |
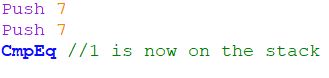 |
CmpNe |
Checks if the top two integers on the stack are not equal |
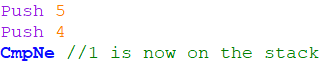 |
CmpGt |
Checks if the first pushed integer is greater than the second pushed integer |
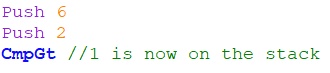 |
CmpGe |
Checks if the first pushed integer is greater than or equal to the second pushed integer |
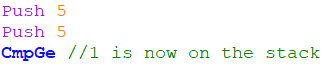 |
CmpLt |
Checks if the first pushed integer is less than the second pushed integer |
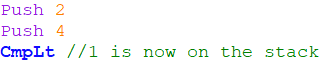 |
CmpLe |
Checks if the first pushed integer is less than or equal to the second pushed integer |
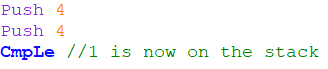 |
fAdd |
Adds the top two floats on the stack |
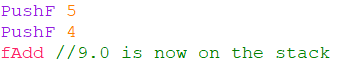 |
fSub |
Subtracts the top two floats on the stack |
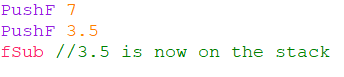 |
fMult |
Multiplies the top two floats on the stack |
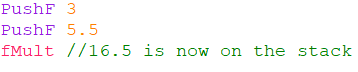 |
fDiv |
Divides the top two floats on the stack (Checks if the divisor is 0) |
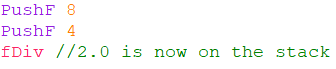 |
fMod |
Performs a modulus on the top two floats on the stack |
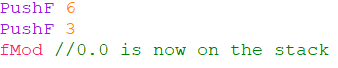 |
fNeg |
Performs a negate on the top float on the stack |
 |
fCmpEq |
Checks if the top two floats on the stack are equal |
 |
fCmpNe |
Checks if the top two floats on the stack are not equal |
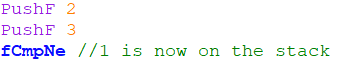 |
fCmpGt |
Checks if the first pushed float is greater than the second pushed float |
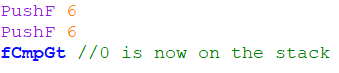 |
fCmpGe |
Checks if the first pushed float is greater than or equal to the second pushed float |
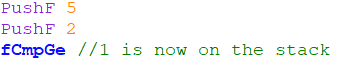 |
fCmpLt |
Checks if the first pushed float is less than the second pushed float |
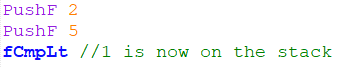 |
fCmpLe |
Checks if the first pushed float is less than or equal to the second pushed float |
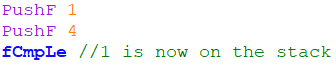 |
vAdd |
Adds the top two vectors on the stack |
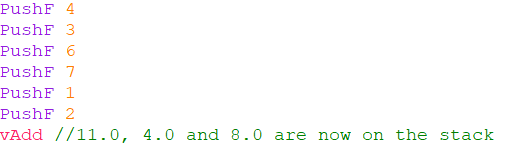 |
vSub |
Subtracts the top two vectors on the stack |
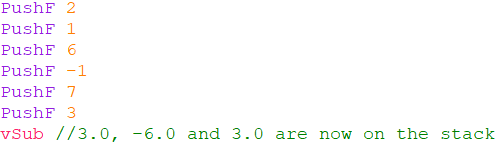 |
vMult |
Multiplies the top two vectors on the stack |
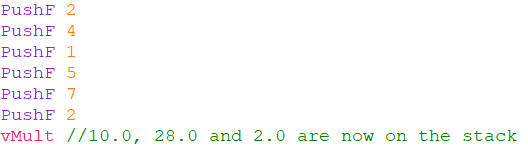 |
vDiv |
Divides the top two vectors on the stack |
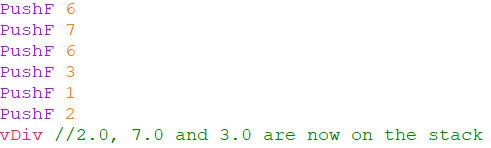 |
vNeg |
Performs a negate on the top vector on the stack |
 |
And |
Performs a bitwise and on the top two integers on the stack |
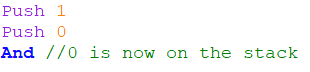 |
Or |
Performs a bitwise or on the top two integers on the stack |
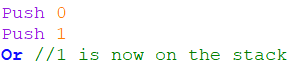 |
Xor |
Performs a bitwise xor operation on the top two integers on the stack |
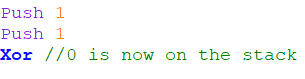 |
ItoF |
Converts the top integer on the stack to a float |
 |
FtoI |
Converts the top float on the stack to an integer |
 |
FtoV |
Loads a float from the stack, then returns a vector of that float |
 |
PushB2 |
Pushes two unsigned 1 byte integers onto the stack |
 |
PushB3 |
Pushes three unsigned 1 byte integers onto the stack |
 |
Push |
Pushes an integer onto the stack |
 |
PushF |
Pushes a float into the stack |
 |
Dup |
Duplicates the top item on the stack |
 |
Drop |
Pops an item off the stack |
 |
CallNative |
Loads param count and the return count from the code buffer, params if any off the stack, then calls the native function |
 |
Function |
Loads the parameter count and the total var count from the code buffer |
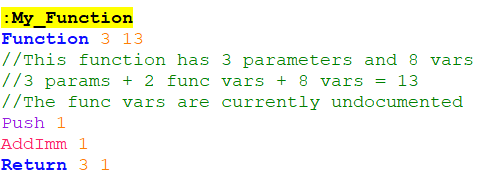 |
Return |
Loads the parameter count and the return variable count from the code buffer |
 |
pGet |
Loads a pointer from the stack, then returns the value of the pointer |
 |
pSet |
Loads a value and a pointer from the stack and stores the value at the pointer |
 |
pPeekSet |
Loads a pointer and a value from the stack, sets the value at the pointer, then keeps the value on the stack |
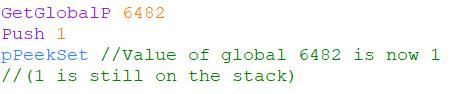 |
ToStack |
Loads the number of items and a pointer from the stack, then returns the items at the pointer |
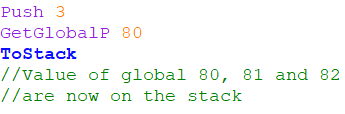 |
FromStack |
Loads the number of items and a pointer from the stack, then loads the items on the stack at the pointer |
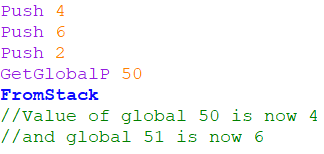 |
GetArrayP |
Loads the array index and the array pointer from the stack, loads the element size from the code buffer, then returns the pointer to the item |
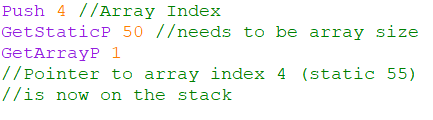 |
GetArray |
Loads the array index and the array pointer from the stack and loads the element size from the code buffer, then returns the value |
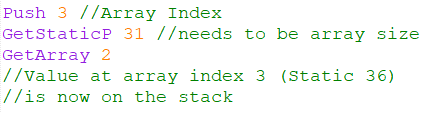 |
SetArray |
Loads the value, array index and array pointer from the stack, and loads the element size from the code buffer, then sets the value at the pointer |
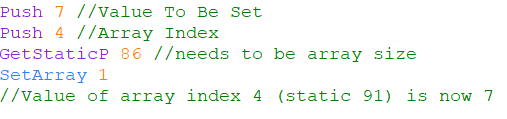 |
GetLocalP |
Loads the variable index of an internal function from the code buffer, then returns the pointer to the index |
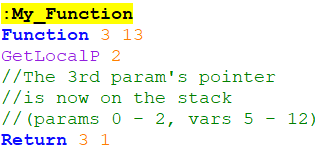 |
GetLocal |
Loads the variable index of an internal function from the code buffer, then returns the value |
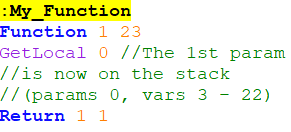 |
SetLocal |
Loads a value off the stack and the variable index of an internal function from the code buffer, then sets the value at the index |
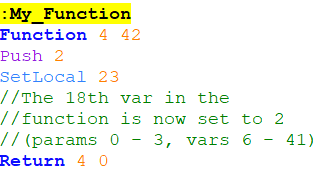 |
GetStaticP |
Loads the index of a static from the code buffer, then returns the pointer to the index |
 |
GetStatic |
Loads the index of a static from the code buffer, then returns the value |
 |
SetStatic |
Loads a value off the stack and the index of a static from the code buffer, then sets the value at the index |
 |
AddImm |
Loads a value from the code buffer and adds it to the value on the stack |
 |
MultImm |
Loads a value from the code buffer and multiplies it to the value on the stack |
 |
GetImmPs |
Loads the pointer and the immediate index from stack, then returns the pointer at the index |
 |
GetImmP |
Loads a pointer from stack and the immediate index from the code buffer, then returns the pointer at the index |
 |
GetImm |
Loads a pointer from stack and the immediate index from the code buffer, then returns the value at the index |
 |
SetImm |
Loads a value and a pointer from the stack, and the immediate index from the code buffer, then sets the value at the index |
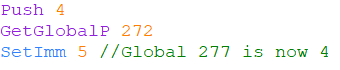 |
GetGlobalP |
Loads the index of a global from the code buffer, then returns the pointer to the index |
 |
GetGlobal |
Loads the index of a global from the code buffer, then returns the value |
 |
SetGlobal |
Loads a value off the stack and the index of a global from the code buffer, then sets the value at the index |
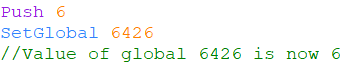 |
Jump |
Loads the jump position from the code buffer and performs an unconditional relative jump |
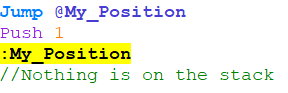 |
JumpFalse |
Loads the jump position from the code buffer and jumps to relative position if top of stack is 0 |
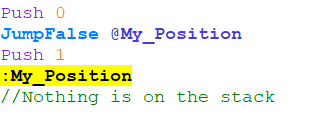 |
JumpTrue |
Loads the jump position from the code buffer and jumps to relative position if top of stack is 1 |
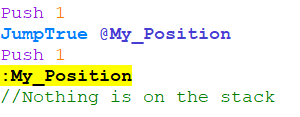 |
JumpNE |
Loads the jump position from the code buffer and jumps to relative position if the top two items on the stack are not equal |
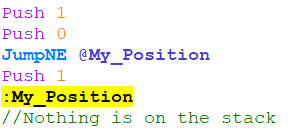 |
JumpEQ |
Loads the jump position from the code buffer and jumps to relative position if the top two items on the stack are equal |
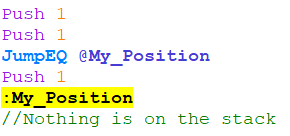 |
JumpLE |
Loads the jump position from the code buffer and jumps to relative position if the first item on the stack is less than or equal to the second |
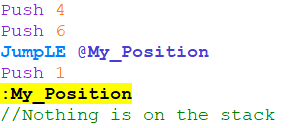 |
JumpLT |
Loads the jump position from the code buffer and jumps to relative position if the first item on the stack is less than the second |
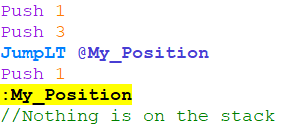 |
JumpGE |
Loads the jump position from the code buffer and jumps to relative position if the first item on the stack is greater than or equal to the second |
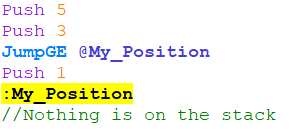 |
JumpGT |
Loads the jump position from the code buffer and jumps to relative position if the first item on the stack is greater than the second |
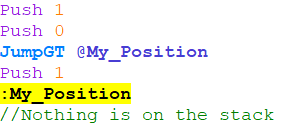 |
Call |
Loads params if any off the stack, then calls to a function in the script |
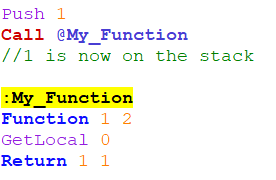 |
Switch |
Loads the index off the stack, case numbers and jump positions from the code buffer, then jumps to the position at case equal to the index |
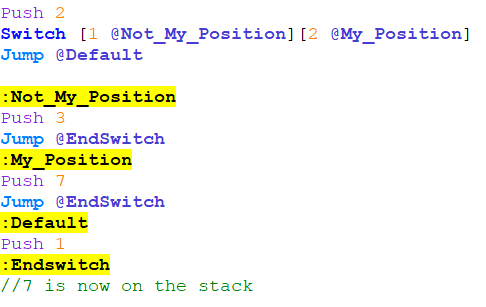 |
PushString |
Pushes a string pointer to the stack |
 |
GetHash |
Loads a value off the stack, then returns a Jenkins hash of the value |
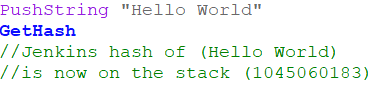 |
StrCopy |
Loads the source string pointer and the dynamic destination string pointer off the stack, and the length from the code buffer, then copies the source into the destination |
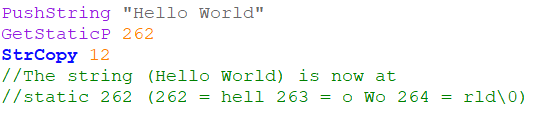 |
ItoS |
Loads a value and a dynamic destination string pointer off the stack, the destination string length off the code buffer, then converts the value into string and places it at the pointer |
 |
StrAdd |
Loads a source string pointer and the dynamic destination string pointer off the stack, and the length from the code buffer, then adds the source onto the destination |
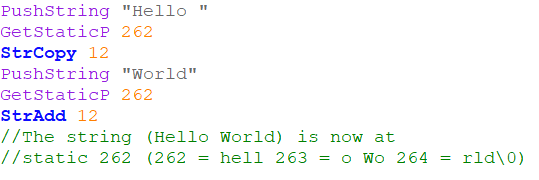 |
StrAddi |
Loads an integer and the destination string pointer off the stack, and the length from the code buffer, then adds the string representation of the integer onto the destination |
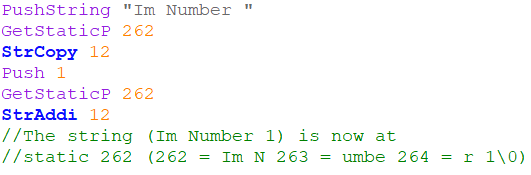 |
MemCopy |
Loads the struct item count, struct size in bytes and the destination pointer the stack, then pops the struct off the stack and sets it into the destination |
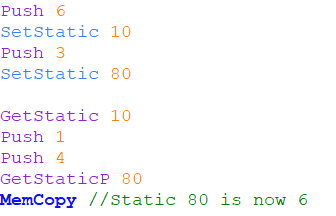 |
Catch |
Sets up a safe area that has the ability to catch errors (Deprecated) |
|
Throw |
Indicates an area that handles a script error relative to the catch opcode (Deprecated) |
|
pCall |
Loads a pointer from the stack and calls to the pointer as a function |
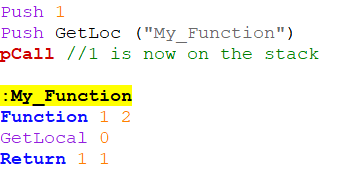 |