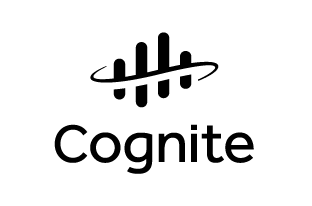
Under development, not recommended for production use cases
CogniteSdk for .NET is a cross platform asynchronous SDK for accessing the Cognite Data Fusion API (v1) using .NET Standard 2.0 that works for all .NET implementations i.e both .NET Core and .NET Framework.
The SDK may be used from both C# and F#.
-
C# SDK: The C# SDK is a fluent API using objects and method chaining. Errors will be raised as exceptions. The API is asynchronous and all API methods returns
Task
and is awaitable usingasync/await
. -
F# SDK: The F# API is written using plain asynchronous functions returning
Task
built on top of the Oryx HTTP handler library.
- Assets
- TimeSeries & DataPoints
- Events
- Files
- Login (partial)
- Raw
- Sequences
- Relationships
- 3D Models
- 3D Files
- 3D Asset Mapping
- Data sets
- SDK Documentation. TBW.
- API Documentation
- API Guide
CogniteSdk is available as a NuGet package. To install:
Using Package Manager:
Install-Package CogniteSdk
Using .NET CLI:
dotnet add package CogniteSdk
The SDK supports authentication through api-keys. The best way to use the SDK is by setting authentication values to environment values:
Using Windows Commands:
setx PROJECT=myprojet
setx API_KEY=mysecretkey
Using Shell:
export PROJECT=myprojet
export API_KEY=mysecretkey
All SDK methods are called with a Client
object. A valid client requires:
API Key
- key used for authentication with CDF.Project Name
- the name of your CDF project e.gpublicdata
.App ID
- an identifier for your application. It is a free text string. Example:asset-hierarchy-extractor
HTTP Client
- The HttpClient that will be used for the remote connection. Having this separate from the SDK have many benefits like using e.g Polly for policy handling.
using CogniteSdk;
var apiKey = Environment.GetEnvironmentVariable("API_KEY");
var project = Environment.GetEnvironmentVariable("PROJECT");
using var httpClient = new HttpClient(handler);
var builder = new Client.Builder();
var client =
builder
.SetAppId("playground")
.SetHttpClient(httpClient)
.SetApiKey(apiKey)
.SetProject(project)
.Build();
// your logic using the client
var query = new Assets.AssetQuery
{
Filter = new Assets.AssetFilter { Name = assetName }
};
var result = await client.Assets.ListAsync(query);
There are examples for both C# and F# in the Playground folder. To play with the example code, you need to set the CDF project and API key as environment variables.
Dependencies for all projects are handled using Paket. To install dependencies:
> dotnet new tool-manifest
> dotnet tool install Paket
> dotnet paket install
This will install the main dependencies and sub-dependencies. The main dependencies are:
- Oryx - HTTP Handlers.
- Oryx.Cognite - Oryx HTTP Handlers for Cognite API.
- Oryx.SystemTextJson - JSON handlers for Oryx
- Oryx.Protobuf - Protobuf handlers for Oryx
- CogniteSdk.Protobuf - Protobuf definitions for Cognite API.
- System.Text.Json - for Json support.
- Google.Protobuf - for Protobuf support.
This project follows https://www.contributor-covenant.org, see our Code of Conduct.
Apache v2, see LICENSE.