Bold is a clone of the e-commerce website, Italic.com. View bold here.
Bold is primarily built with the a combination of following four technologies:
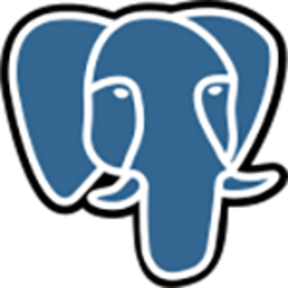
Update cart item quantities or delete items within the cart show page.
// update quantity in cart function
const updateQuantity = (field) => {
if (field === "add") {
cartProduct.quantity ++;
dispatch(editCartProduct(cartProduct.cart_id, cartProduct));
} else {
cartProduct.quantity --;
dispatch(editCartProduct(cartProduct.cart_id, cartProduct));
}
}
<div className="cart__actions">
<div className="cart__actions__quantity">
// button checks if quantity falls below 1 then delete item
<div className="cart__actions__quantity__quantity-button"
onClick={ (cartProduct.quantity > 1) ?
() => updateQuantity("subtract") :
() => dispatch(deleteCartProduct(cartProduct.cart_id, cartProduct.id))
}
>-</div>
<div className="cart__actions__quantity">{cartProduct.quantity}</div>
<div className="cart__actions__quantity__quantity-button"
onClick={() => updateQuantity("add")}>+</div>
</div>
// provide multiple ways to remove products from cart
<div className="cart__delete">
<img src="https://italic.com/static/icons/clear-x.svg"
alt="delete product"
className="cart__delete__button"
onClick={() => dispatch(deleteCartProduct(cartProduct.cart_id, cartProduct.id))} />
<div className="cart__delete__link"
onClick={() => dispatch(deleteCartProduct(cartProduct.cart_id, cartProduct.id))}>
Remove
</div>
</div>
</div>
Toggle easily between different images for any given product.
<div className="Outter_Photos_Container">
<div className="Inner_Photos_Container">
// left side photo thumbnails
// when clicked, the photo index state is updated and a new product image is rendered
<div className="Thumbnail_Display">
{
photos.map((photoUrl, idx) => (
<div
className="Thumbnail"
key={idx}
onClick={() => { setIndex(idx) }}
>
<img src={photoUrl} alt={product.product.title}/>
</div>
))
}
</div>
// main image displayed here
<div className="Product_Photos">
<div className="Product_Photos_Separator">
<img className="Product_Photo" src={photos[index]} alt={product.product.title} />
</div>
</div>
</div>
</div>
- Checkout
- Promt user for review upon checkout
- Reviews
- Rank newest reviews first
- Add timeStamps to reviews
- Add image to review
- User account
- View order history
- Search
- Search by category