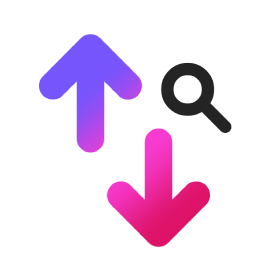
Caution
This library is not stable, and the API may change. It is not advised to use it in production projects.
Inspektor is an HTTP inspection library for Ktor. It allows you to view HTTP requests and responses, including basic information, headers, and bodies. Please note that this library is not stable, and the API may change. Users are not advised to use it in production projects.
Here's an introductory article for those who are interested.
Add the following dependency to your build.gradle.kts
file:
dependencies {
implementation("com.gyanoba.inspektor:inspektor:latest-version")
}
To use Inspektor, install the plugin in your HttpClient
configuration:
import io.ktor.client.HttpClient
import io.ktor.client.plugins.api.install
import io.ktor.client.request.get
import com.gyanoba.inspektor.Inspektor
// For Android this is enough
val client = HttpClient {
install(Inspektor)
}
suspend fun apiCall() {
client.get("http://example.com")
}
For ios you need to add the following -lsqlite3
to the Other Linker flags under Build Settings.
See more details here
For Desktop platforms, you need to specify the APPLICATION_ID using setApplicationId
before using
Inspektor.
This is used to determine the location to store the database file.
import data.db.setApplicationId
fun main() {
setApplicationId("com.example.myapp")
// ...
}
You can customize Inspektor using the InspektorConfig
object. Here are the available options:
level
: Specifies the logging level. Available options areLogLevel.NONE
,LogLevel.INFO
,LogLevel.HEADERS
, andLogLevel.BODY
.maxContentLength
: Sets the maximum content length for logging request and response bodies.filter
: Allows you to filter log messages for calls matching a predicate.sanitizeHeader
: Allows you to sanitize sensitive headers to avoid their values appearing in the logs.
install(Inspektor) {
level = LogLevel.HEADERS
maxContentLength = 100_000
filter { request -> request.url.host.contains("example.com") }
sanitizeHeader { header -> header == "Authorization" }
}
This feature is meant to be used for testing purposes. It allows you to override the request and response bodies. To use this feature, open the overrides page from the menu. Add new overrides by specifying matchers for the request; then select the action to perform, i.e. override the request or response. Only the values specified are replaced in the request or response. Empty values are ignored.
Request.Response.Overriding.mp4
You can easily add new overrides by clicking on the Edit icon in the Transactions List screen. Default override with original info gets created which you can then modify to get your desired result.
Inspektor provides a UI to view the logs. You can access it by invoking openInspektor
function.
This opens up a new activity in Android, a bottom sheet in iOS, and a new window in the Desktop.
On Android you can also open the UI by clicking on the generated notifications.
- Request-Response overriding functionality
- Pause and allow editing Request and Response
- HAR export for detailed analysis
- More HTTP client support (OkHttp maybe?)
This project is licensed under the MIT License. See the LICENSE file for details.
This project is inspired by Chucker - An HTTP inspector for Android & OkHttp. It borrows many ideas (and some code 😉) from the project.
This library is not stable, and the API may change. Users are not advised to use it in production projects.