This program is a simple two-dimensional physics engine simulating inelastic collisions between balls and their surroundings. Term project for CPSC 210 (Software Construction) at the University of British Columbia.
What does this application do?
This project is able to simulate a 2 dimensional physical environment with object collisions under the effects of gravity and friction. In addition, objects may be created, saved, and loaded by a user, with specifiable physical attributes.
Who will use it?
This project is primarily intended to be used by a younger demographic of students in an educational setting, where they can "get their hands dirty" and toy around with various physical phenomena.
Why did I decide on this project idea?
I thought that it was a challenging task to translate theoretical concepts I learned from other classes into concrete code which can accurately explain real-world phenomena, particularly that of momentum. Not only will this help me gain a deeper understanding of these concepts, but will also allow me to practice object-oriented programming techniques to solve problems.
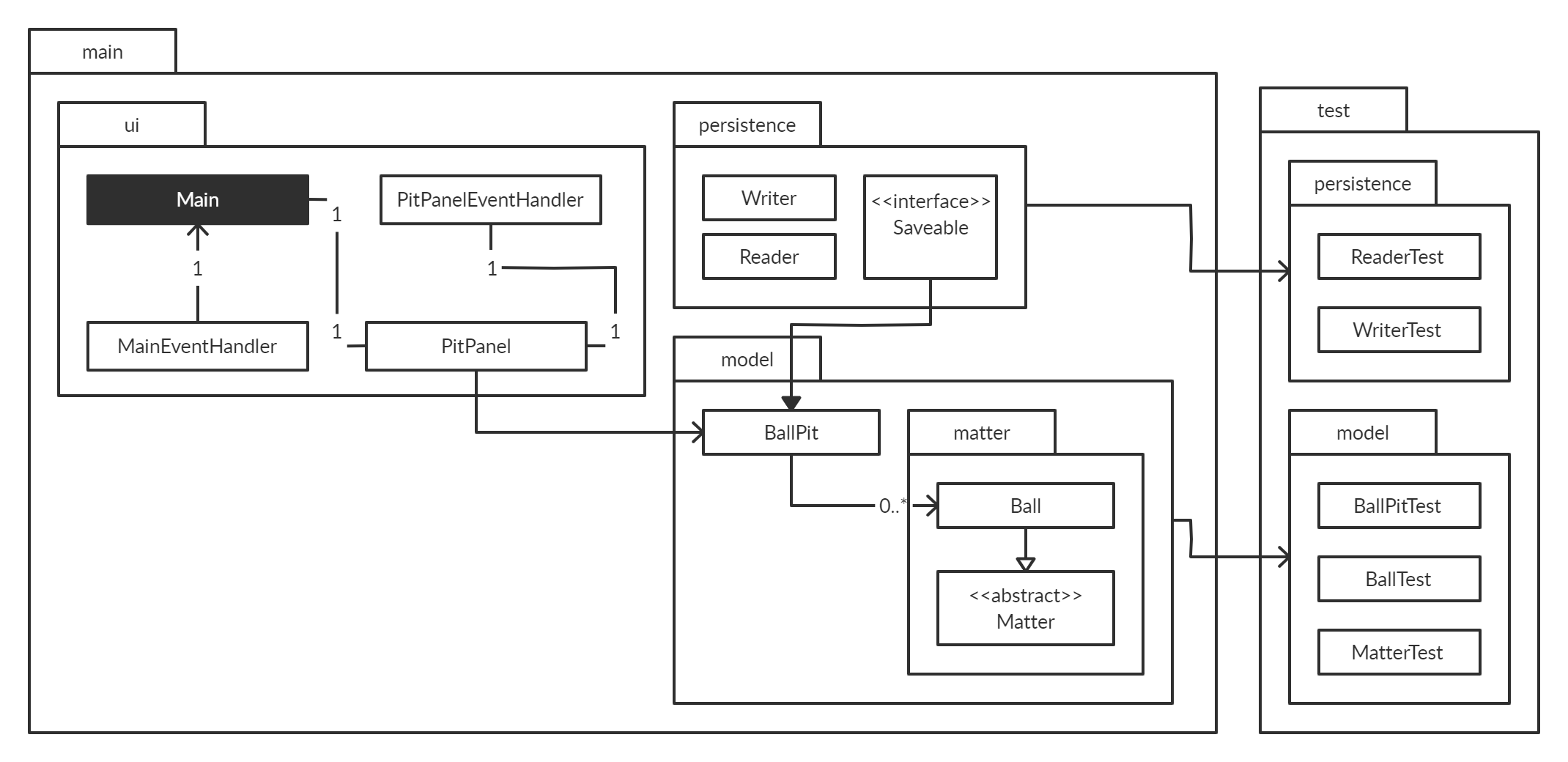
- Matter is an abstract class holding generic information regarding objects within the environment (e.g. mass, speed, and position).
- Ball is a specific subclass of Matter, with properties specific to a ball (e.g. radius)
- BallPit is an aggregate class of all of Matter objects, and environment characteristics (e.g. width, height).
As a user, some of the things I want the program to do are...
- see the balls move
- add balls to the BallPit
- delete balls from the BallPit
- see the balls interact with each other and the environment
As a user, I want to features that allow me to...
- save the current state of the sandbox locally
- load another sandbox's state
- prompt me to save when exiting the sandbox
In order to refactor the program from a console application to an application with a GUI, I implemented a Main class to handle displaying the main menu with data persistence options, and a PitPanel class to display the instantiated BallPit object, along with corresponding controls.
I chose to re-design a class such that it is robust. Particularly, I decided to use this on the Ball class, such that balls with impossible mass and radius cannot be constructed. The methods which use this robust design are the constructors which create each ball.
I chose to fix poor cohesion regarding the Main class. In this particular class, one of the main issues is that in addition to running the main program (specifically ball pit related functions), this class had also included methods responsible for mouse events and key events. To solve this lack of cohesion, I made a separate class to handle mouse events and key events called MainEventHandler.
A similar problem existed for the PitPanel class, which should be responsible only for rendering the ball pit onto the JFrame. However, there was poor cohesion, as PitPanel was also responsible for handling user input, in the form of key events and mouse events. To fix this poor cohesion, I made another class called PitPanelEventHandler in order to handle key and mouse events from the user while the ball pit is being rendered.