QuickSms library will help you achieve the following:
- Send SMS easily.
- Check Account Balance.
- Get Delivery Report.
Simply register at quicksms1.com and you are good to go!
Add this in your build.gradle
implementation 'com.emmanuelkehinde:quicksms:1.0.0'
Initialize QuickSms with your Username and Password
QuickSms quickSms = new QuickSms.Builder()
.setUsername("YOUR_EMAIL")
.setPassword("YOUR_PASSWORD")
.build();
Create an Sms
Instance
Sms sms = new Sms.Builder()
.setSender("Tester")
.setRecipient("08000000000")
.setMessage("This is just a test")
.build();
Using the sms
instance as an argument
quickSms.sendSms(sms)
.addOnSuccessListener(new OnSuccessListener<SendSmsResult>() {
@Override
public void onSuccess(SendSmsResult result) {
showToastMessage(result.getMessageId());
}
})
.addOnFailureListener(new OnFailureListener() {
@Override
public void onFailure(QuickSmsException e) {
showToastMessage(e.getMessage());
}
});
Sms sms = new Sms.Builder()
...
...
.setRecipient("08000000000,08000000001,08000000002")
.build();
Sms sms = new Sms.Builder()
...
...
.isDndSupported(true)
.build();
Sms sms = new Sms.Builder()
...
...
.schedule("2018-10-01 12:30:00")
.build();
quickSms.getAccountBalance()
.addOnSuccessListener(new OnSuccessListener<GetAccountBalanceResult>() {
@Override
public void onSuccess(GetAccountBalanceResult result) {
showToastMessage(result.getBalance().toString());
}
})
.addOnFailureListener(new OnFailureListener() {
@Override
public void onFailure(QuickSmsException e) {
showToastMessage(e.getMessage());
}
});
Using messageID
as an argument
quickSms.getDeliveryReport("45795")
.addOnSuccessListener(new OnSuccessListener<GetDeliveryReportResult>() {
@Override
public void onSuccess(GetDeliveryReportResult result) {
showToastMessage(result.getStatus());
}
})
.addOnFailureListener(new OnFailureListener() {
@Override
public void onFailure(QuickSmsException e) {
showToastMessage(e.getMessage());
}
});
If this library helps you in anyway, show your love ❤️ by putting a ⭐ on this project ✌️; or you can buy me a coffee 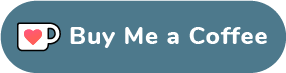
Copyright (C) 2018 Emmanuel Kehinde
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
All pull requests are welcome, make sure to follow the contribution guidelines when you submit pull request.