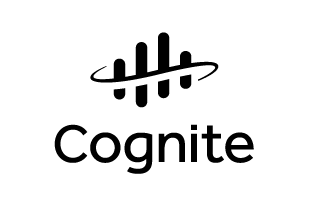
The Cognite js library provides convenient access to the Cognite API from applications written in client- or server-side JavaScript.
The SDK supports authentication through api-keys (for server-side applications) and bearer tokens (for web applications).
Install the package with yarn:
$ yarn add @cognite/sdk
or npm
$ npm install @cognite/sdk --save
const { CogniteClient } = require('@cognite/sdk');
import { CogniteClient } from '@cognite/sdk';
The SDK is written in native typescript, so no extra types needs to be defined.
import { CogniteClient } from '@cognite/sdk';
async function quickstart() {
const client = new CogniteClient({ appId: 'YOUR APPLICATION NAME' });
client.loginWithOAuth({
project: 'publicdata',
});
const assets = await client.assets
.list()
.autoPagingToArray({ limit: 100 });
}
quickstart();
For more details about SDK authentication see this document.
Also, more comprehensive intro guide with a demo app can be found here
const { CogniteClient } = require('@cognite/sdk');
async function quickstart() {
const client = new CogniteClient({ appId: 'YOUR APPLICATION NAME' });
client.loginWithApiKey({
project: 'publicdata',
apiKey: 'YOUR_SECRET_API_KEY',
});
const assets = await client.assets
.list()
.autoPagingToArray({ limit: 100 });
}
quickstart();
Samples are in the samples/ directory. The samples' README.md has instructions for running the samples.
See this guide on how to migrate from version 1.x.x
to version 2.x.x
.
There is one integration test that requires a api key. In order to run this, you need an api key for the cognitesdk-js
tenant. Talk to any of the contributors or leave an issue and it'll get sorted. Travis will run the test and has its own api key.
Set the environment variable COGNITE_PROJECT
to cognitesdk-js
and COGNITE_CREDENTIALS
to your api-key.
Run all tests:
$ yarn
$ yarn test
Set the environment variable REVISION_3D_INTEGRATION_TEST=true
to run 3D revision integration tests.
We use jest
to run tests, see their documentation for more information.
The library follow Semantic Versioning.
Contributions welcome! See the code of conduct.
Our releases are fully automated. Only basic steps are needed:
- Create a new branch
- Commit changes (if any) and remember about proper commit messages
- Create a new pull request
- A new version will be published when the PR is merged
You can find it here.