A password generator compiled as Wasm for use on the CLI, in a custom element, or as a module.
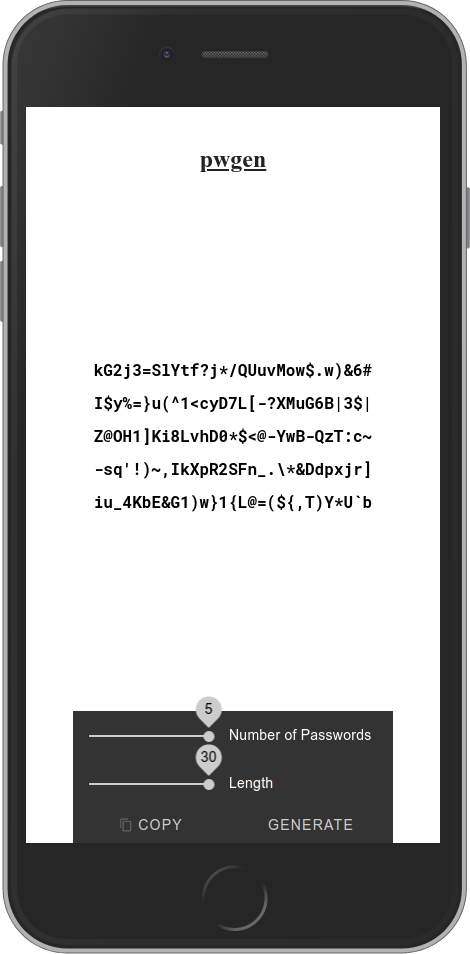
npx pwgen -sy 20 1
npm i -g pwgen
pwgen -sy 20 1
wapm install kherrick/pwgen
wapm run pwgen -sy 20 1
Use in HTML (demo)
<x-pwgen
composed
flags="-sy"
length="20"
number="1"
></x-pwgen>
<script type="module">
import 'https://unpkg.com/pwgen'
document.addEventListener(
'x-pwgen-handle-password',
({ detail }) => {
console.log(detail.msg)
}
)
</script>
npm i pwgen
import React from 'react';
import 'pwgen';
const App: React.FC = () => {
return (
<x-pwgen></x-pwgen>
)
}
npm i pwgen
Within the component:
import { Component, OnInit } from '@angular/core';
import { pwgen } from 'pwgen';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent implements OnInit {
password: string = '';
ngOnInit() {
pwgen({
arguments: ['-sy', '20', '1'],
print: (password: string) => (
this.password = password
),
});
}
}
Within the template:
<ng-container>{{ password }}</ng-container>
npm i pwgen
const pwgen = require('pwgen')
const flags = '-1sy'
const length = '20'
const number = '10'
pwgen({
arguments: [ flags, length, number ],
print: stdout => {
console.log(`Password: ${stdout}`)
}
})
Download pwgen.wbn
git clone https://github.com/kherrick/pwgen \
&& cd pwgen \
&& git submodule update --init --recursive
npm start
npm run build
Show command line usage and available options (flags):
npx pwgen --help
Usage: pwgen [ OPTIONS ] [ pw_length ] [ num_pw ]
Options supported by pwgen:
-c or --capitalize
Include at least one capital letter in the password
-A or --no-capitalize
Don't include capital letters in the password
-n or --numerals
Include at least one number in the password
-0 or --no-numerals
Don't include numbers in the password
-y or --symbols
Include at least one special symbol in the password
-r <chars> or --remove-chars=<chars>
Remove characters from the set of characters to generate passwords
-s or --secure
Generate completely random passwords
-B or --ambiguous
Don't include ambiguous characters in the password
-h or --help
Print a help message
-H or --sha1=path/to/file[#seed]
Use sha1 hash of given file as a (not so) random generator
-C
Print the generated passwords in columns
-1
Don't print the generated passwords in columns
-v or --no-vowels
Do not use any vowels so as to avoid accidental nasty words