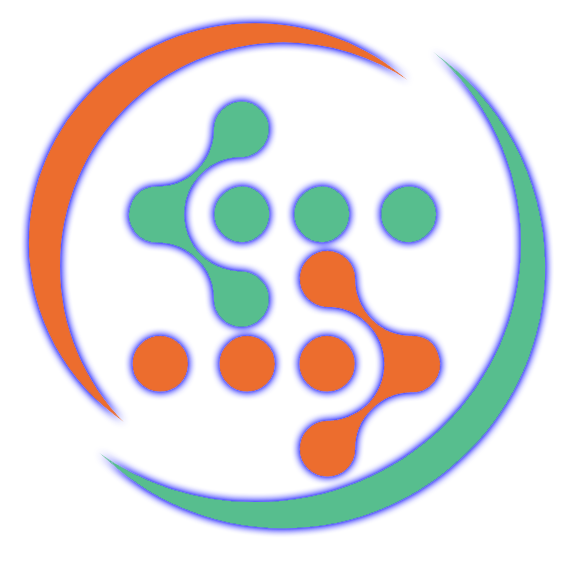
Speak, hear, and see with one line of javascript, or REST API.
Powered by astica
Vision Demo
·
Hearing Demo
·
Voice Demo
·
GPT-S Demo
These demonstrations require an API key for the astica.ai cognitive API. You can register and get your key instantly.
<script src="https://astica.ai/javascript-sdk/2024-01-31/astica.api.js"></script>
<script>
asticaAPI_start('API KEY HERE'); //only needs to be called once.
//Simple Vision:
asticaVision('Image URL or Base64'); //simple computer vision
asticaVision('Image URL or Base64', 'Description,Faces,Objects'); //with options:
//Simple Listen:
asticaListen('https://www.astica.org/endpoint/ml/inputs/audio/sample_1.wav');
//Simple Voice:
asticaVoice('Hi! How are you doing today?');
//Simple GPT-S:
asticaGPT('What color is an apple?');
</script>
Get started by including the astica.api.js within your project.
-
Get your API Key at https://astica.ai
-
Add the astica javascript API to your project:
<script src="https://astica.ai/javascript-sdk/2024-01-31/astica.api.js"></script>
-
Authenticate:
asticaAPI_start('API KEY HERE'); //only needs to be called once.
-
Using asticaVision:
<script src="https://astica.ai/javascript-sdk/2024-01-31/astica.api.js"></script> <script> asticaAPI_start('API KEY HERE'); //run at least once //Example 1: asticaVision('Image URL', 'Objects'); //simple computer vision //Example 2: asticaVision('Image URL', 'Description,Faces,Objects'); //with options: //Example 3: //advanced with parameters: asticaVision( '1.0_full', //modelVersion: 1.0_full, 2.0_full 'IMAGE URL', //Input Image 'Description,Moderate,Faces', //or 'all' your_astica_CallBack, //Your Custom Callback function ); //Set Your Custom Callback Function function your_astica_CallBack(data) { if(typeof data.error != 'undefined') { alert(data.error); } console.log(data); //view all data } </script>
-
Using asticaListen with remote File:
<script src="https://astica.ai/javascript-sdk/2024-01-31/astica.api.js"></script> <script> //simple usage function asticaListen_Sample_simple() { asticaListen('https://www.astica.org/endpoint/ml/inputs/audio/sample_1.wav'); } setTimeout(function() { asticaAPI_start('API KEY HERE'); //run at least once asticaListen_Sample_simple(); }, 2000); //with parameters: function asticaListen_Sample() { var astica_modelVersion = '1.0_full'; var astica_modelInput = 'https://www.astica.org/endpoint/ml/inputs/audio/sample_1.wav'; //With default callback: asticaListen(astica_modelVersion, astica_modelInput); //With custom callback function: asticaListen(astica_modelVersion, astica_modelInput, your_astica_CallBack); } function your_astica_CallBack(data) { if(typeof data.error != 'undefined') { alert(data.error); } console.log(data); //view all data } setTimeout(function() { asticaAPI_start('API KEY HERE'); //run at least once asticaListen_Sample(); }, 1000); </script>
-
Using asticaListen with local File:
<script src="https://astica.ai/javascript-sdk/2024-01-31/astica.api.js"></script> <script> var asticaTranscribeFile_input = document.getElementById('astica_ML_voice_input'); var asticaTranscribeFile_localData; document.addEventListener("DOMContentLoaded", () => { asticaTranscribeFile_input.addEventListener("change", function () { asticaTranscribeFile = asticaTranscribeFile_input.files[0]; }); }); function asticaVoice_transcribeFile_test() { asticaAPI_start(document.getElementById("astica_ML_apikey").value); //only needs to be called once. asticaListen_file('1.0_full', asticaTranscribeFile, your_astica_CallBack); } function your_astica_CallBack(data) { if(typeof data.error != 'undefined') { alert(data.error); return; } console.log(data); } //view all data </script>
-
Using asticaVoice:
<script src="https://astica.ai/javascript-sdk/2024-01-31/astica.api.js"></script> <script> asticaAPI_start('API KEY HERE'); //only needs to be called once. //Simple usage: asticaVoice('Hi! How are you doing today?'); //Specify a voice id: asticaVoice('Hi! How are you doing today?', 5); //With custom callback: asticaVoice_speak('Hi! How are you doing today?', 5, your_astica_CallBack); function your_astica_CallBack(data) { if(typeof data.error != 'undefined') { alert(data.error); return; } } //With function and paramaters: function asticaVoice_example(string) { asticaVoice( '1.0_full' string, 'en-US', 0, your_astica_CallBack ); } setTimeout(function() { asticaAPI_start('API KEY HERE'); //only needs to be called once. asticaVoice_example('Hi! How are you doing?'); }, 1000); </script>
-
Using asticaGPT:
<script src="https://astica.ai/javascript-sdk/2024-01-31/astica.api.js"></script> <script> asticaAPI_start('API KEY HERE'); //only needs to be called once. //Simple Usage: asticaGPT_generate("Write a sentence about apples:") //Simple Usage with Token Limit: asticaGPT_generate("Write a sentence about apples:", 10) //With Token Limit: asticaGPT_generate("Write a sentence about apples:", 10) //Advanced: var asticaGPT_params = { temperature:document.getElementById("astica_ML_temperature").value, top_p:document.getElementById("astica_ML_top_p").value, think_pass:document.getElementById("astica_ML_think_pass").value, stop_sequence:document.getElementById("astica_ML_stop_sequence").value, stream_output: document.getElementById("astica_ML_stream_output").value } asticaGPT_generate( document.getElementById("astica_ML_version").value, document.getElementById("astica_ML_gpt_input").value, document.getElementById("astica_ML_tokens_max").value, asticaGPT_params ); //default asticaGPT callbacks function asticaGPT_generateComplete(data){ console.log(data); if(typeof data.error != 'undefined') { alert(data.error); } $('#your_div').val(data.output) } function asticaGPT_generatePreview(data){ console.log(data); if(typeof data.error != 'undefined') { alert(data.error); return; } $('#your_div').val(data.output) } //Plug and play example: function asticaGPT_example(string, tokens) { asticaGPT_generate(string, tokens) } setTimeout(function() { asticaAPI_start('API KEY HERE'); //only needs to be called once. asticaGPT_example('Write a sentence about apples:', 55); //max 55 tokens }, 1000); </script>