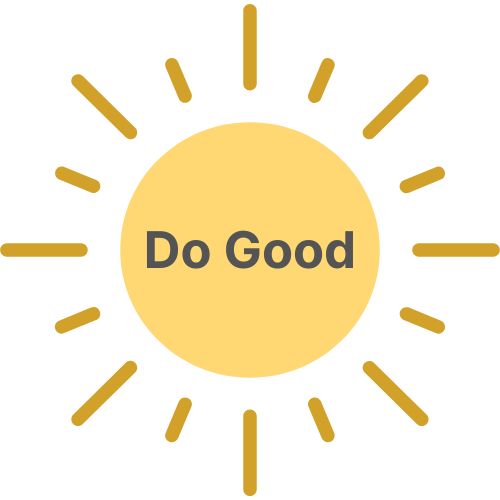
Find the GOOD in the world.
With the constant bombardment of negative news, it's easy to think there is no good left in the world. Do Good aims to increase the good by connecting people with opportunities to complete random acts of kindness in an approachable and fun way.
Table of Contents
☀️ Production Website
☀️ Backend Service
☀️ Front End Repository
Do Good was created by Back End students at Turing School of Software and Design as their consultancy project.
The mission of Do Good is to provide users with convenient access to positivity and an opportunity to be part of the good in the world.
Users simply click the "Find a Random Act of Kindness" button and are presented with 3 random acts to choose from. Once chosen, they schedule the "good deed" and it gets added to their dashboard. Users are able to invite others to join in on the fun as well as add it to their calendars because, let's be real, life can get hectic.
For visitors who aren't ready to jump into completing an act, they can browse our library of kind act photos for the feel-goods.
If you'd like to demo this API on your local machine:
- Ensure you have the prerequisites
- Clone this repo:
git clone git@github.com:do-good-2211/do_good_api.git
- Navigate to the root folder:
cd do_good_api
- Run:
bundle install
- Run:
rails db:{create,migrate}
- Inspect the
/db/schema.rb
and compare to the 'Schema' section below to ensure migration has been done successfully - Run:
rails s
- Visit http://localhost:3000/
- Ruby Version 3.1.1
- Rails Version 7.0.4.x
- Bundler Version 2.4.9
To test the entire spec suite, run bundle exec rspec
.
All tests should be passing.
Happy path, sad path, and edge testing were considered and tested. When a request cannot be completed, an error object is returned.
Error Object
{
"errors": [
{
"status": "404"
"title": "Invalid Request",
"detail": [
"Couldn't find User with 'id'="
]
}
]
}
GET "/api/v1/good_deeds"
Response:
{
"data": [{
"id": “1”,
"type": “good_deeds",
"attributes":{
"name": "Deed",
"media_link": "www.image.com/picture",
"time": "2000-01-01T16:00:00.000Z",
"date": "2023-01-01"
}
}]
}
GET "/api/v1/random_acts"
Response:
{
"data": {
"id": null,
"type": “random_acts",
"attributes":{
"deed_names": ["Deed 1", "Deed 2", "Deed 3"]
}
}
}
GET "/api/v1/users"
Response:
{
"data": [{
"id": “1”,
"type": “users",
"attributes": {
"name": "Joe",
"role": "user",
"email": "joe@email.com",
"good_deeds": {
"id": 1,
"type": "good_deed",
"attributes": {
"name": "Help Others",
"date": "2023-01-01",
"time": "2000-01-01T16:00:00.000Z",
"status": "Completed",
"notes": "Worth it!",
"media_link": "https://image.com",
"host_id": 1,
"host_name": "Jean-Luc Picard",
"attendees": [{
"id": 2,
"name": "William Riker",
"email": "number2@gmail.com",
"role": "user",
"created_at": "2023-04-19T16:11:24.633Z",
"updated_at": "2023-04-19T16:11:24.633Z",
"uid": "22022",
"provider": "Google"
}
]
}
}
}
}]
}
GET "/api/v1/users/:user_id"
Response
{
"data": {
"id": “1”,
"type": “users",
"attributes":{
"name": "Sam",
"role": "user",
"email": "sam@email.com",
"good_deeds": [{
"data": {[
"id": “1”,
"type": “good_deed",
"attributes":{
"name": "Deed",
"media_link": "www.image.com/picture",
"notes”: “ex description”,
“date”: "02-02-2024",
"time": "10:00,
"status": "Completed",
"host_id": "1",
"host_name": "Sam",
"attendees": [{
"id": 2,
"name": "William Riker",
"email": "number2@gmail.com",
"role": "user",
"created_at": "2023-04-19T16:11:24.633Z",
"updated_at": "2023-04-19T16:11:24.633Z",
"uid": "22022",
"provider": "Google"
}]
}
]
}
}]
}
}
}
GET "/api/v1/users/:user_id/good_deeds/:good_deed_id"
Response:
{
"data": {
"id": "1",
"type": "good_deed",
"attributes": {
"name": "Deed",
“date”: "02-02-2024",
"time": "10:00,
"status": "in progress",
"attendees": [{
"id": 1,
"name": "William",
"email": "william@email.com",
"created_at": "2023-04-19T16:11:24.633Z",
"updated_at": "2023-04-19T16:11:24.633Z",
"uid": "22022",
"provider": "Google"
}]
}
}
}
POST "/api/v1/users/:user_id/good_deeds"
Request Body:
{
"name": "Deed",
"date": "02-02-2024",
"time": "10:00",
"host_id": "2",
"status": "In Progress",
"attendees": []
}
Response:
{
"data": {
"id": "6",
"type": "good_deeds_create",
"attributes": {
"name": "Deed",
"media_link": null,
"notes": null,
"date": "2024-02-02",
"time": "2000-01-01T10:00:00.000Z",
"status": "In Progress",
"host_id": 1
}
}
}
PATCH "/api/v1/users/:user_id/good_deeds/:good_deed_id"
Request Body:
{
"name": "Deed",
"date": "02-02-2024",
"time": "10:00",
"notes": "Stuff and things",
"media_link": "picture.jpg"
}
Response:
{
"data": {
"id": "1",
"type": "good_deed",
"attributes": {
"name": "Deed",
"date": "2024-02-02",
"time": "2000-01-01T10:00:00.000Z",
"status": "Completed",
"notes": "Stuff and things",
"media_link": "picture.jpg",
"host_id": 1,
"host_name": "Jean-Luc Picard",
"attendees": [
{
"id": 2,
"name": "William Riker",
"email": "number2@gmail.com",
"role": "user",
"created_at": "2023-04-19T16:11:24.633Z",
"updated_at": "2023-04-19T16:11:24.633Z",
"uid": "22022",
"provider": "Google Baby!"
}
]
}
}
}
DELETE "/api/v1/users/:user_id/good_deeds/:good_deed_id"
Response
Status: 204
View these endpoints in Postman
The BoredAPI was consumed to generate the random acts of kindness.
Google Calendar API was used to create a calendar event on the user's Google Calendar.
Google Cloud Platform was used to allow users to sign in with their Google account.
Google OAuth
Signing in to the Do Good app is made simple by allowing users to sign in with Google.
More information on the gem used for this(omniauth-google-oauth2
) can be found here
Amazon Web Services was used to allow users to upload photos for their completed good deeds.
Amazon S3 Cloud Object Storage
By including amazon's web serfice for storage, we can allow users to upload their pictures which are then saved as objects in a "bucket".
More information on the gem used for this(aws-sdk-s3
) can be found here
Twilio SendGrid was used to send an email to the user after they create a good deed
Rafactor/Changes
- Pull other APIs
- - Locally available volunteer opportunities
- Refactor Serializers
- - Specifcally the good deeds serializers
![]() |
![]() |
![]() |
![]() |
![]() |
![]() |
---|---|---|---|---|---|
Huy Phan | Jasmine Hamou | Kara Jones-Hofmann | Matt Enyeart | Melony Erin Franchini | Mike Dao |
Collaborator | Collaborator | Collaborator | Collaborator | Collaborator | Project Manager |
GitHub | GitHub | GitHub | GitHub | GitHub | GitHub |