The PayButton helps make the integration of card acceptance into your app easy.
You simply provide the merchant information you receieve from PaySky to the payment SDK. The PayButton displays a ready-made view that guides the merchant through the payment process and shows a summary screen at the end of the transaction.
This project uses cocoapods for dependencies management. If you don't have cocoapods installed in your machine, or are using older version of cocoapods, you can install it in terminal by running command sudo gem install cocoapods
or brew install cocoapods
.
For more information go to Cocoapods.org
- Close Xcode project.
- Create a Podfile to your project. From the terminal navigate to project location then use next command
pod init
- Add the pod to your Podfile: Open Podfile with any text editor tool then add the next line inside "target 'PayButton' do "scoop
pod 'PayButtonIOS', :git => 'https://github.com/payskyCompany/PayButtonIOS.git', :branch => 'master'
- Open the terminal and run
pod deintegrate
pod install
To avoid pods DEPLOYMENT_TARGET
errors add the next lines to the end of podFile
post_install do |installer|
installer.pods_project.targets.each do |target|
target.build_configurations.each do |config|
config.build_settings['IPHONEOS_DEPLOYMENT_TARGET'] = '13.0'
end
end
end
- Disable
USER_SCRIPT_SANDBOXING
- Reopen _.xcworkspace.
- Navigate to targets and select your app name.
- Navigate to Build Settings
- Search for USER_SCRIPT_SANDBOXING and set it to NO.
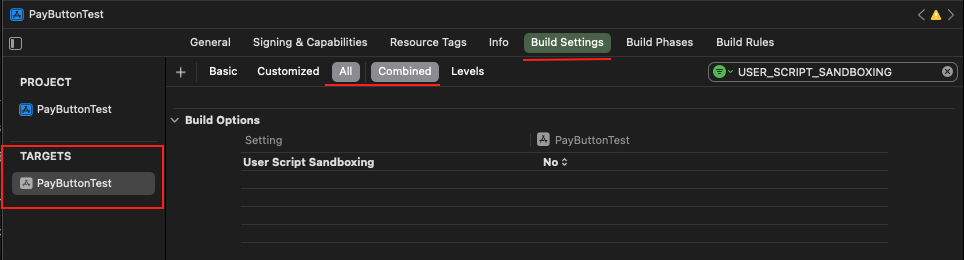
If you have this error :
Multiple commands produce '/Users/paysky106/Library/Developer/Xcode/
DerivedData/TestPayButton-ghdftwdswnoxgexfnpfxqmwsgah/Build/Products/
Debug-iphonesimulator/TestPayButton.app/Assets.car'
Place disable_input_output_paths: true in Podfile, to skip optimisation and always download resources from Pods cleanly every time, since it is not copying bundled assets during build. Adding extra overhead to build doesn't seem optimal, and leads to bad experience during local App development
# Podfile
# platform :ios, '13.0'
install! 'cocoapods', :disable_input_output_paths => true
- Before deploying your project live, you should get a merchant ID and terminal ID provided by PaySky.
- You should keep your merchant ID and terminal ID secured in your project, encrypt them before save them in project.
In order to use the SDK you should use a production merchantId, terminalId and secure_hash key provided by PaySky.
In the class you want to intiate the payment from, you should import the framework
import PayButtonIOS
After the import, create a new instance from PayButton
let paymentViewController = PaymentViewController(
merchantId: "merchantId", //Mandatory
terminalId: "terminalId", //Mandatory
amount: 100.0, //Mandatory - provide the amount and currency with it's decimal factor
currencyCode: 0, //Mandatory - Provided by PaySky
secureHashKey: "secure_hash",//Mandatory - Provided by PaySky
trnxRefNumber: "", //Optinal (remove it if not use), Provided by PaySky
customerId: "", //Optinal (remove it if not use), Provided by PaySky
customerMobile: "", //Optinal (remove it if not use), Provided by PaySky
customerEmail: "", //Optinal (remove it if not use), Provided by PaySky
isProduction: false //Choose the needed inviroment
)
Then confirm to PaymentDelegate :-
paymentViewController.delegate = self // Payment Delegate
paymentViewController.pushViewController()
In order to create transaction callback in delegate PaymentDelegate, implement delegate on your ViewController.
extension ViewController: PayButtonDelegate {
func finishedSdkPayment(_ response: PayButtonIOS.PayByCardReponse) {
if response.success == true {
print("Transaction completed successfully")
print(response.networkReference ?? "") // reference number of transaction.
} else {
print("Transaction failed")
print(response.message ?? "") // response error
}
}
}
PaySky Company