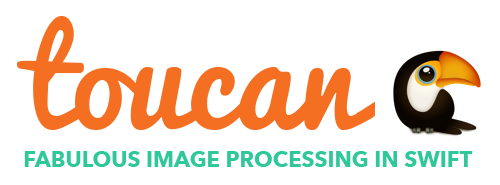

Toucan is a Swift library that provides a clean, quick API for processing images. It greatly simplifies the production of images, supporting resizing, cropping and stylizing your images.
- Easy and smart resizing
- Elliptical and rounded rect masking
- Mask with custom images
- Chainable image processing stages
- 100% Unit Test Coverage - once image loading in XCTests are fixed!
- Add crop options for crop location & faces
- Add enhancement filters to beautify images
- Add stylize filters
- Add rotation and flip support
- Lazy evaluation of image contexts to prevent having to create and close multiple contexts during method chaining
As of version 0.4, Toucan only supports Swift 2. Use version 0.3.x for the latest Swift 1.2 compatible release
- Include the
Toucan
framework by dragging it into your project and import the library in your code using import Toucan
Currently there are issues loading the library using pod 'Toucan'
which is pending changes from Cocoapods.
Toucan provides two methods of interaction - either through wrapping an single image within a Toucan instance, or through the static functions, providing an image for each invocation. This allows for some very flexible usage.
Create an instance wrapper for easy method chaining:
let resizedAndMaskedImage = Toucan(image: myImage).resize(CGSize(width: 100, height: 150)).maskWithEllipse().image
Or, using static methods when you need a single operation:
let resizedImage = Toucan.Resize.resizeImage(myImage, size: CGSize(width: 100, height: 150))
let resizedAndMaskedImage = Toucan.maskWithEllipse(resizedImage)
Resize the contained image to the specified size. Depending on what fitMode
is supplied, the image may be clipped, cropped or scaled.
Toucan(image: myImage).resize(size: CGSize, fitMode: Toucan.Resize.FitMode)
or
Toucan.Resize.resizeImage(image: UIImage, size: CGSize, fitMode: FitMode = .Clip) -> UIImage
FitMode drives the resizing process to determine what to do with an image to make it fit the given size bounds.
Example |
Mode |
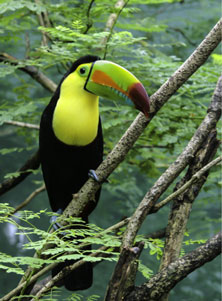 |
Clip Mode
Toucan.Resize.FitMode.Clip Resizes the image to fit within the width and height boundaries without cropping or distorting the image.
Toucan(image: portraitImage).resize(CGSize(width: 500, height: 500), fitMode: Toucan.Resize.FitMode.Clip).image |
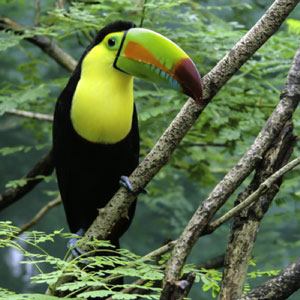 |
Crop Mode
Toucan.Resize.FitMode.Crop Resizes the image to fill the width and height boundaries and crops any excess image data.
Toucan(image: portraitImage).resize(CGSize(width: 500, height: 500), fitMode: Toucan.Resize.FitMode.Crop).image |
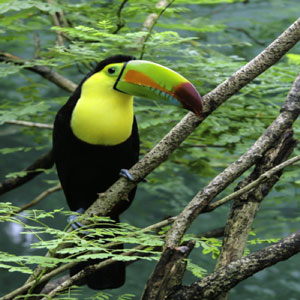 |
Scale Mode
Toucan.Resize.FitMode.Scale Scales the image to fit the constraining dimensions exactly.
Toucan(image: portraitImage).resize(CGSize(width: 500, height: 500), fitMode: Toucan.Resize.FitMode.Scale).image |
Alter the original image with a mask; supports ellipse, rounded rect and image masks.
Example |
Function |
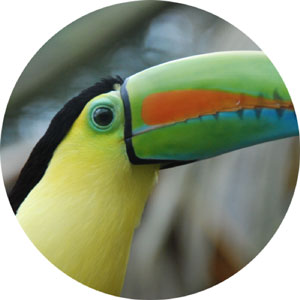 |
Mask the given image with an ellipse. Allows specifying an additional border to draw on the clipped image. For a circle, ensure the image width and height are equal!
Toucan(image: myImage).maskWithEllipse().image or
Toucan.Mask.maskImageWithEllipse(myImage) -> UIImage |
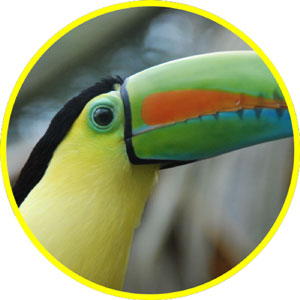 |
When specifying a border width, it is draw on the clipped image.
Toucan(image: myImage).maskWithEllipse(borderWidth: 10, borderColor: UIColor.yellowColor()).image or
Toucan.Mask.maskImageWithEllipse(myImage, borderWidth: 10, borderColor: UIColor.yellowColor()) -> UIImage |
Example |
Function |
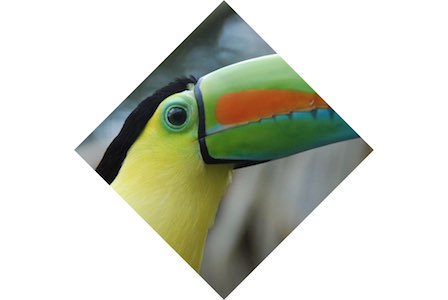 |
Mask the given image with a path. The path will be scaled to fit the image correctly!
path.moveToPoint(CGPointMake(0, 50))
path.addLineToPoint(CGPointMake(50, 0))
path.addLineToPoint(CGPointMake(100, 50))
path.addLineToPoint(CGPointMake(50, 100))
path.closePath()
Toucan(image: myImage).maskWithPath(path: path).image or
Toucan.Mask.maskImageWithPath(myImage, path: path) -> UIImage |
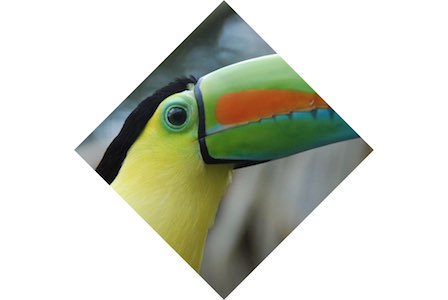 |
Mask the given image with a path provided via a closure. This allows you to construct your path relative to the bounds of the image!
Toucan(image: myImage).maskWithPathClosure(path: (rect: CGRect) -> (UIBezierPath)).image or
Toucan.Mask.maskImageWithPathClosure(myImage, path: (rect: CGRect) -> (UIBezierPath)) -> UIImage |
Example |
Function |
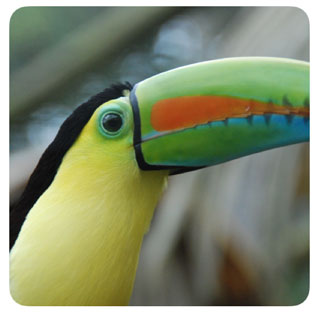 |
Mask the given image with a rounded rectangle border. Allows specifying an additional border to draw on the clipped image.
Toucan(image: myImage).maskWithRoundedRect(cornerRadius: 30).image or
Toucan.Mask.maskImageWithRoundedRect(myImage, cornerRadius: 30) -> UIImage |
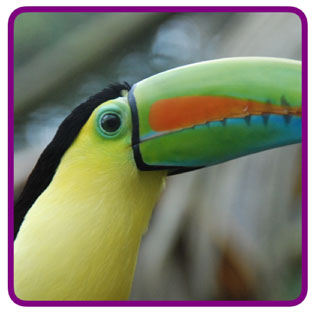 |
When specifying a border width, it is draw on the clipped rounded rect.
Toucan(image: myImage).maskWithRoundedRect(cornerRadius: 30, borderWidth: 10, borderColor: UIColor.purpleColor()).image or
Toucan.Mask.maskImageWithRoundedRect(myImage, cornerRadius: 30, borderWidth: 10, borderColor: UIColor.purpleColor()) -> UIImage |
Example |
Function |
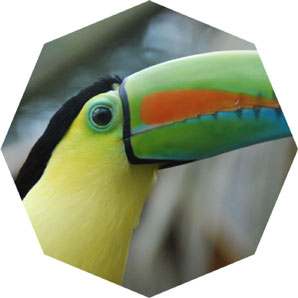 |
Mask the given image with another image mask. Note that the areas in the original image that correspond to the black areas of the mask show through in the resulting image. The areas that correspond to the white areas of the mask aren’t painted. The areas that correspond to the gray areas in the mask are painted using an intermediate alpha value that’s equal to 1 minus the image mask sample value.
Toucan(image: myImage).maskWithImage(maskImage: octagonMask).image or
Toucan.Mask.maskImageWithImage(myImage, maskImage: octagonMask) -> UIImage |
Example images used under Creative Commons with thanks to:
- Please fork this project
- Implement new methods or changes in the
Toucan.swift
file.
- Write tests in the
ToucanTests
folder.
- Write appropriate docs and comments in the README.md
- Submit a pull request.
Raise an Issue or hit me up on Twitter @gavinbunney
Toucan is released under an MIT license. See LICENSE for more information.