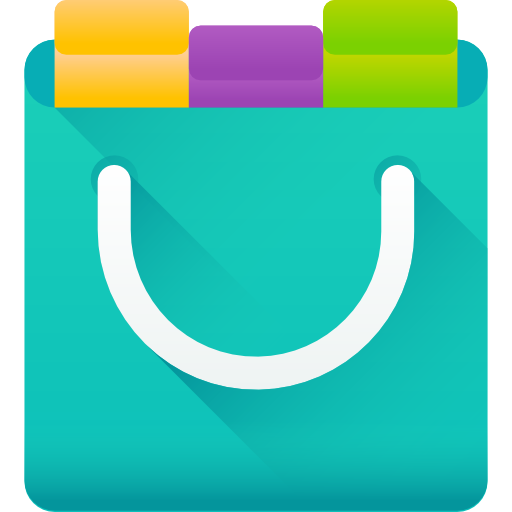
🛒 Shop App
A simple Shop App to browse, add your own products, add products to cart and later order them.
Download APK & Source Code
Universal Release
arm64
x64
armabi
Source Code (zip)
Source Code (tar.gz)
👨🎓 Things I learned through this project
State Management
- Working with Providers, listeners and notifiers.
- Using a Provider class in another Provider class.
- Using Consumers for more optimizations i.e. rebuilding only the builder function or a part of widget.
- Using Provider to provide data application-wide or multiple widgets, OR
- Using Stateful Widget instead of Provider when the state only affect a widget or its child widget.
Working with User Input & Forms
- Handling and validating the User Input.
- Showing dialogs to interact with Users
Working with HTTP Requests & Error Handling
- Using Http requests like post, get, patch, etc to do CRUD operations.
- Using Future & Asynchronous Fn with async/await.
- Error Handling, using try-catch(-finally) or catcherror.
Working with User Authentication
- Using Firebase Authentication to Log in/out, sign up, etc.
- Managing Auth token locally using Shared Preferences.
- Using the ProxyProvider & attaching the Token to outgoing HTTP requests.
- Using the FutureBuilder to show different widgets based on the future state.
- Automatically Logging In/Out functionality.
Animation
- Using built-in animations like Hero Animation, various Transition Animations, etc.
- Using AnimatedBuilder/AnimatedContainer to animate a widget without rebuilding it.
- Implementing Custom Route Transitions.
Dependencies Used
http: ^0.13.4
intl: ^3.2.0
provider: ^10.1.0
shared_preferences: ^2.0.15
📱 App UI
Click here to expand
Login/Signup Screen | Home Screen | Product Detail Screen |
---|---|---|
App Drawer | Filter PopUp | Cart Screen |
Orders Screen | Manage Products Screen | Edit Product Screen |
Directory Structure
shop_app
|
|-- assets
| |-- fonts
| | |-- Anton-Regular.ttf
| | |-- Lato-Bold.ttf
| | `-- Lato-Regular.ttf
| |
| `-- images
| |-- background.png
| |-- clock.png
| |-- light-1.png
| |-- light-2.png
| `-- shop_app.png
|
|-- lib
| |-- helpers
| | `-- custom_route.dart
| |
| |-- models
| | `-- http_exception.dart
| |
| |-- providers
| | |-- auth.dart
| | |-- cart.dart
| | |-- orders.dart
| | |-- product.dart
| | `-- products.dart
| |
| |-- screens
| | |-- auth_screen.dart
| | |-- cart_screen.dart
| | |-- edit_product_screen.dart
| | |-- orders_screen.dart
| | |-- product_detail_screeen.dart
| | |-- products_overview_screen.dart
| | |-- splash_screen.dart
| | `-- user_products_screen.dart
| |
| |-- widgets
| | |-- app_drawer.dart
| | |-- badge.dart
| | |-- cart_item.dart
| | |-- order_item.dart
| | |-- product_item.dart
| | |-- products_grid.dart
| | `-- user_product_item.dart
| |
| `-- main.dart
|
|-- pubspec.yaml
|
`-- README.md
- If you don't have Flutter SDK installed, please visit official Flutter site.
- Fetch latest source code from master branch.
rajput-hemant@arch:~$ git clone https://github.com/rajput-hemant/shop-app
- Run the app with Android Studio or VS Code. Or the command line:
rajput-hemant@arch:~$ flutter pub get
rajput-hemant@arch:~$ flutter run