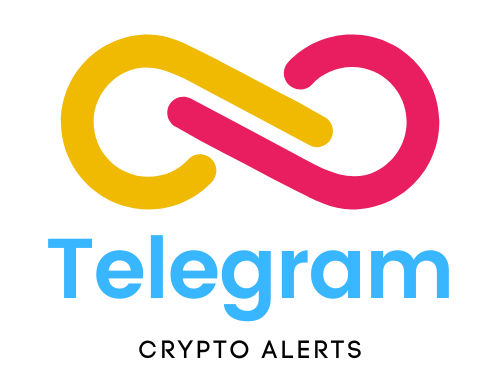
Python software that facilitates alerts on cryptocurrency price movements and technical indicators through Telegram using their open-source API, and optionally email as well.
About the Project • Getting Started • Bot Commands • Add Indicators • Contribute • Contact
⚠️ Disclaimer⚠️ Due to recent changes with the Binance regulations, some IPs may be blocked from accessing the Binance API. If you are experiencing issues with the bot, please try using a VPN or proxy service (e.g. NordVPN, IPVN, CyberGhost).
The primary goal of Telegram Crypto Alerts is to be a lightweight, intuitive, and modular cryptocurrency price alert bot for the Telegram messaging client.
The bot utilizes Telegram's simple chat interface to provide users the following features:
- Get live crypto pair prices from Binance, and receive alerts on price movements like above, below, % change, and 24 hour % change
- Receive alerts on crypto technical indicators like RSI, MACD, Bollinger Bands, MA, SMA, and EMA.
- The bot has the capacity to support any technical indicator that is available on Taapi.io, but only these are shipped from the start. See How to Add Technical Indicators for more information.
- Optionally receive dynamic HTML-styled email alerts using the SendGrid API
- Configure bot access with a full suite of administrator commands
- Invite your friends to use the bot!
- Add additional users with their own unique alerts and configuration.
- Optional state and configuration data - Use a local JSON database or configure a MongoDB server!
Alternatively, follow the steps below to set it up on your local machine.
-
Clone the repository:
git clone https://github.com/hschickdevs/Telegram-Crypto-Alerts.git
-
CD into the repository root directory:
cd /Telegram-Crypto-Alerts
-
Install the required Python package dependencies:
pip install -r requirements.txt
-
Ensure that you have Python 3.9+ installed. If not, you can download here. The syntax is dependent on features added in this recent version.
-
If you haven't already, create a telegram bot using BotFather and get the bot token.
-
In the https://t.me/BotFather telegram chat:
- type /mybots
- -> @yourbot
- -> Edit Bot
- -> Edit Commands
- Paste and send the contents of
commands.txt
into the chat
-
Ensure that you are in the repository root directory
cd Telegram-Crypto-Alerts
-
Set your environment variables or create a
.env
file in the root directory with the following:TELEGRAM_BOT_TOKEN=123456789:ABCDEFGHIJKLMNOPQRSTUVWXYZ # Your telegram bot token TAAPIIO_APIKEY=123456789.ABCDEFGHIJKLMNOPQRSTUVWXYZ # Your TAAPI.IO API key TAAPIIO_APIKEY2=123456789.ABCDEFGHIJKLMNOPQRSTUVWXYZ # Alternate TAAPI.IO key for the telegram message handler (OPTIONAL) SENDGRID_APIKEY=your_apikey # Your SendGrid API key for automated email alerts (OPTIONAL) ALERTS_EMAIL=your_email # The email from which you would like alerts to be sent from (must be registered on SendGrid)
If the SENDGRID_APIKEY and ALERTS_EMAIL variables are not set, only Telegram alerts will be available. The bot uses these email credentials to send email alerts using SendGrid. You can sign up here for free.
Email alerts are disabled in the configuration by default, but if you did set a Sendgrid API key and email you can enable email alerts using the
/setconfig send_email_alerts=true
command once you start the bot.The alert emails are dynamically built from the
src/templates/email_template.html
file. -
(OPTIONAL) MongoDB Setup & Configuration
As of 7/16/2023, this bot now supports MongoDB as a database backend to enable data persistence. If you'd like to use a MongoDB server to store your data, instead of the local JSON database default, follow the steps below:
First, set the
USE_MONGO_DB
config variable toTrue
in the static configuration file:/bot/config.py
Next, you will need to create a MongoDB Atlas account. You can sign up here.
Once you have created an account, you will need to create a new databse using the Altas UI: https://www.mongodb.com/basics/create-database#option-1
You will need to locate the following:
- Your
database connection string
- Your
database name
- Your
database collection name
All of the documents required for the program's backend are stored in the same collection. The documents are differentiated by the
user_id
field. Theuser_id
field is the telegram user id of the user that the document belongs to.You will then need to set the following environment variables or add them to your
.env
file:MONGODB_CONNECTION_STRING=your_connection_string # The URI for your MongoDB server MONGODB_DATABASE=your_database # The name of the database to use MONGODB_COLLECTION=your_collection # The name of the database collection to use
- Your
-
(IMPORTANT) Run the setup script using the
setup.py
file to create your admin account:# Windows: python setup.py --id YOUR_TELEGRAM_USER_ID # or py setup.py --id YOUR_TELEGRAM_USER_ID # Mac/Linux: python3 setup.py --id YOUR_TELEGRAM_USER_ID
This script will set up the local json data files for your user configuration and alerts. If you don't know how to get your telegram user id, use the command:
python setup.py --help
Note: If you want to add another user as an admin on the bot, you can run the setup script again with the
--id
argument and the additional user's telegram user id. -
Run the
bot
module using:# Windows: python -m bot # or py -m bot # Mac/Linux: python3 -m bot
You can either run this script on your local machine, or use a cloud computing service like Heroku or PythonAnywhere.
-
/viewalerts # Returns all active alerts /indicators # View the list of all available types of simple and technical indicators with their detailed descriptions. """Create new alert for simple indicators ONLY (see /indicators)""" /newalert <BASE/QUOTE> <INDICATOR> <COMPARISON> <TARGET> <optional_ENTRY_PRICE> # - BASE/QUOTE: The base currency for the alert (e.g. BTC/USDT) # - INDICATOR: Currently, the only available simple indicator is "PRICE" # - COMPARISON: The comparison operator for the alert (ABOVE, BELOW, PCTCHG, or 24HRCHG) # - TARGET: The target value for the alert (For PCTCHG and 24HRCHG, use % form e.g. 10.5% = 10.5) # - optional_ENTRY_PRICE: If using the "PCTCHG" comparison opperator, you can specify this as an alternate entry price to the current price for calculating percentage changes. # Creates a new active simple indicator alert with the given parameters. # E.g. /newalert BTC/USDT PRICE PCTCHG 10.0 1200 """Create new alert for technical indicators ONLY (see /indicators)""" /newalert <BASE/QUOTE> <INDICATOR> <TIMEFRAME> <PARAMS> <OUTPUT_VALUE> <COMPARISON> <TARGET> # - BASE/QUOTE: The base currency for the alert (e.g. BTC/USDT) # - INDICATOR: The ID for the technical indicator (e.g. RSI) # - TIMEFRAME: The desired time interval for the indicator (Options: 1m, 5m, 15m, 30m, 1h, 2h, 4h, 12h, 1d, or 1w) # - PARAMS: No-space-comma-separated list of param=value pairs for the indicator (E.g. period=10,stddev=3) (Use "default" to skip passing params and use default values) (See /indicators for available params) # - OUTPUT_VALUE: The desired output value to monitor (See /indicators for available output values) # - COMPARISON: The comparison operator for the alert (Options: ABOVE or BELOW) # - TARGET: The target value of <OUTPUT_VALUE> for the alert to trigger # Creates a new active technical indicator alert with the given parameters. # E.g. /newalert ETH/USDT BBANDS 1d default valueUpperBand ABOVE 1500 /cancelalert <base/quote> <index> # Cancels the pair alert at the given index (use /viewalerts <base/quote> to see the indexes) # e.g. /cancelalert BTC/USDT 1
-
/getprice <base/quote> # Get the current pair price from Binance /priceall # Get the current pair price for all pairs with active alerts. /getindicator <BASE/QUOTE> <INDICATOR> <TIMEFRAME> <PARAMS> # - BASE/QUOTE: The base currency for the alert (e.g. BTC/USDT) # - INDICATOR: The ID for the technical indicator (e.g. BBANDS) # - TIMEFRAME: The desired time interval for the indicator (Options: 1m, 5m, 15m, 30m, 1h, 2h, 4h, 12h, 1d, or 1w) # - PARAMS: No-space-comma-separated list of param=value pairs for the indicator (E.g. period=10,stddev=3) (use "default" to use the default values for the indicator) # Get the current value(s) of a technical indicator # E.g. /getindicator ETH/USDT BBANDS 1d default
-
/viewconfig # Returns the current general configuration for the bot /setconfig <key>=<value> <key>=<value> # Modify individual configuration settings # e.g. /setconfig send_email_alerts=True # You can change multiple settings by separating them with a space /channels VIEW/ADD/REMOVE <telegram_channel_id>,<telegram_channel_id> # VIEW - Returns the current list of Telegram channels in which to send price alerts # ADD - Adds each of the telegram channel ids (separated by a comma) to the channel registry. The <telegram_channel_id> parameter can be either a user's telegram id or a channel's telegram id # REMOVE - Removes each of the telegram channel ids (separated by a comma) from the channel registry # Interact with your channels alert output configuration # e.g. /channels ADD 123456789,987654321 /emails VIEW/ADD/REMOVE <email@email.com>,<email@email.com> # VIEW - Returns the current list of emails in which to send price alerts. If the send_email_alerts config is set to False, emails will not be sent. # ADD - Adds each of the emails (separated by a comma) to the email registry. # REMOVE - Removes each of the emails (separated by a comma) from the channel registry # Interact with your email alert output configuration # e.g. /channels ADD 123456789,987654321
-
/admins VIEW/ADD/REMOVE <telegram_user_id>,<telegram_user_id> # VIEW - Returns the current list of admins from the registry # ADD - Adds each of the telegram user ids (separated by a comma) to the admin registry # REMOVE - Removes each of the telegram user ids (separated by a comma) from the admin registry # Interact with the bot's administrator list # e.g. /admins ADD -123456789,-987654321 /whitelist VIEW/ADD/REMOVE <telegram_user_id>,<telegram_user_id> # VIEW - Returns the current whitelist # ADD - Adds each of the telegram user ids (separated by a comma) to the whitelist # REMOVE - Removes each of the telegram user ids (separated by a comma) from the whitelist # Interact with the bot's whitelist # e.g. /whitelist ADD -123456789,-987654321 /getlogs # Returns the current process logs
As stated previously, the bot is designed to be easily extensible. View the currently available indicators by using the /indicators
command on the bot. If your indicator is not listed, head over to taapi.io/indicators and find the indicator you want to add. You can add any technical indicator that is supplied by taapi.io by following the steps below:
-
Shut the bot down if it is currently running using
CRTL+C
in the terminal window. -
Open the
/util/add_indicators.ipynb
file using jupyter notebook.If you don't have jupyter installed, see this guide: https://jupyter.org/install
-
Make a new cell, and use the
db.add_indicator
function to add the indicator to the database:View the previous examples of how the existing indicators were added to the database using the
db.add_indicator
function (seeTADatabaseClient.add_indicator
in/bot/indicators.py
)The usage of the
db.add_indicator
function is as follows:db.add_indicator( indicator_id # Name/abbreviation of the endpoint as shown on taapi.io (e.g. BBANDS, MACD, MA) name # The full name of the indicator on taapi.io (e.g. Bollinger Bands, Moving) endpoint # the endpoint for the indicator in the following format: https://api.taapi.io/{indicator_id}?secret={api_key}&exchange=binance reference_url # The url to the taapi.io documentation for the indicator params # A list of tuples to specify additional parameters for the indicator: (param_name, param_description, default_value) output # A list of output values for the indicator, as shown on taapi.io (e.g. upperBand, middleBand, lowerBand) )
-
endpoint - Must match the format shown above. The bot automatically adds the
symbol
andinterval
required parameters. Additional parameters can be added using theparams
. -
params - The
param_name
must match the name of the parameter as shown on taapi.io. Theparam_description
is a short description of the parameter (used in the /indicators command). Thedefault_value
is your custom default value for the parameter.Using the following screenshot below as an example:
The params list would look like the following:
[ ("optInFastPeriod", "Fast period length", 12), ("optInSlowPeriod", "Slow period length", 26), ("optInSignalPeriod", "Signal smoothing", 9), ]
-
output - The
output
list must match the output values as shown on taapi.io.Additionally, the output value must be directly accessible from the API response as keys in a dictionary. For example, the following response would be valid:
{ "valueMACD": 737.4052287912818, "valueMACDSignal": 691.8373005221695, "valueMACDHist": 45.56792826911237 }
Because of this, parameters such as
backtracks
are restricted because they turn the response into a list of dictionaries. The bot is not designed to handle this type of response. The following response would NOT be valid:[ { "valueMACD": 979.518807843051, "valueMACDSignal": 893.54139321284, "valueMACDHist": 85.977414630211, "backtrack": 0 }, { "valueMACD": 949.7317001653792, "valueMACDSignal": 872.0470395552873, "valueMACDHist": 77.6846606100919, "backtrack": 1 }, ]
You may need to DYOR to ensure that the parameters that you are configuring will result in a valid response.
-
Contributions are always welcome! To contribute to the project, please do one of the following:
- Create a new issue and describe your idea/suggestion in detail
- Create a pull request
- Fork the project
- Create a branch for your new edits (E.g. new-indicator)
- Implement and test your changes (test, test, test!)
- Submit your pull request
I am actively maintaining this project, and I will respond to any issues or pull requests as soon as possible.
Please Star ⭐ the project if it helps you so that visibility increases to help others!
If you have any questions, feel free to reach out to me on Telegram.
- Scale to multiple unique user configurations~~
- Build infrastructure to integrate indicators from taapi.io