
List a collection of items in a horizontally scrolling view. A scaling factor controls the size of the items relative to the center.

- iOS 8.0+
- Xcode 9.0+
Just add the RACarousel directory to your project.
or use CocoaPods with Podfile:
pod 'RACarousel'
or Carthage users can simply add Mantle to their Cartfile
:
github "Ramotion/ra-carousel"
or just drag and drop the RACarousel directory to your project
-
Create a custom view that will be used as a carousel item.
-
Create a view controller or container view that handles datasource and delegate responses for the contained Carousel.
2.1) Add the selection of a delegate and datasource to your Carousel control.
@IBOutlet var carousel : RACarousel! {
set {
_carousel = newValue
_carousel.delegate = self
_carousel.dataSource = self
}
get {
return _carousel
}
}```
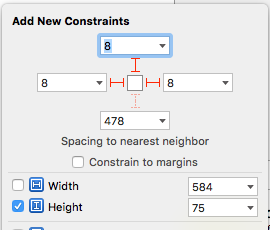
3) Select how you want the carousel to operate based on the control variables specified below :

Your result should be something like this picture:
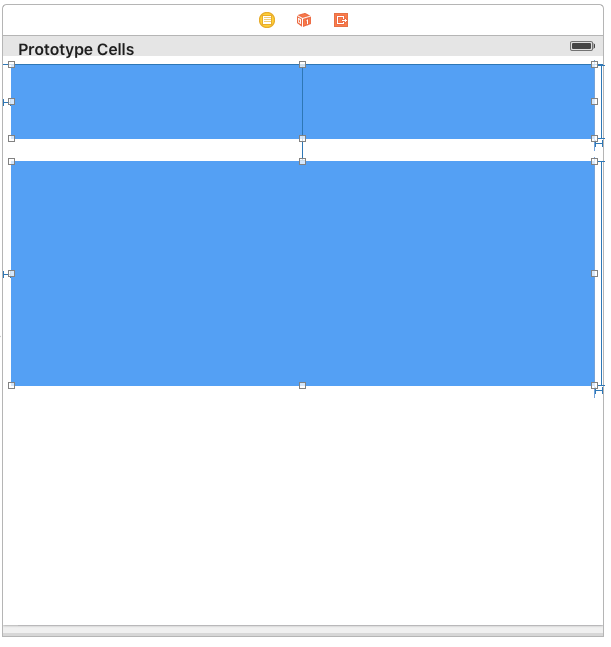
4) Specify the control variables in interface builder.
4.1) Add the delegate method to provide values to the Carousel from the container view or controller.
That's it, the Carousel is good to go.
``` swift
fileprivate struct C {
struct CellHeight {
static let close: CGFloat = *** // equal or greater foregroundView height
static let open: CGFloat = *** // equal or greater containerView height
}
}
5.2) Add property for calculate cells height
var cellHeights = (0..<CELLCOUNT).map { _ in C.CellHeight.close }
5.3) Override method:
override func tableView(tableView: UITableView, heightForRowAtIndexPath indexPath: NSIndexPath) -> CGFloat {
return cellHeights[indexPath.row]
}
5.4) Added code to method:
override func tableView(tableView: UITableView, didSelectRowAtIndexPath indexPath: NSIndexPath) {
guard case let cell as FoldingCell = tableView.cellForRowAtIndexPath(indexPath) else {
return
}
var duration = 0.0
if cellIsCollapsed {
cellHeights[indexPath.row] = Const.openCellHeight
cell.unfold(true, animated: true, completion: nil)
duration = 0.5
} else {
cellHeights[indexPath.row] = Const.closeCellHeight
cell.unfold(false, animated: true, completion: nil)
duration = 0.8
}
UIView.animateWithDuration(duration, delay: 0, options: .CurveEaseOut, animations: { _ in
tableView.beginUpdates()
tableView.endUpdates()
}, completion: nil)
}
5.5) Control if the cell is open or closed
override func tableView(tableView: UITableView, willDisplayCell cell: UITableViewCell, forRowAtIndexPath indexPath: NSIndexPath) {
if case let cell as FoldingCell = cell {
if cellHeights![indexPath.row] == C.cellHeights.close {
foldingCell.selectedAnimation(false, animated: false, completion:nil)
} else {
foldingCell.selectedAnimation(true, animated: false, completion: nil)
}
}
}
- Add this code to your new cell class
override func animationDuration(itemIndex:NSInteger, type:AnimationType)-> NSTimeInterval {
// durations count equal it itemCount
let durations = [0.33, 0.26, 0.26] // timing animation for each view
return durations[itemIndex]
}
Create foregroundView and containerView from code (steps 2 - 3) look example: Folding-cell-programmatically
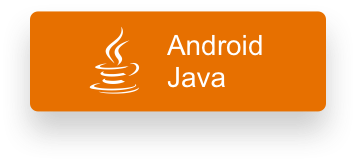
Carousel is released under the MIT license. See LICENSE for details.
This library is a part of a selection of our best UI open-source projects.
If you use the open-source library in your project, please make sure to credit and backlink to www.ramotion.com
Try this UI component and more like this in our iOS app. Contact us if interested.

