This library provides a simple way to create barcodes using only the Python standard lib. The barcodes are created as SVG objects.
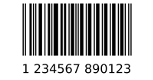
Please report any bugs at https://github.com/WhyNotHugo/python-barcode/issues
- Setuptools/distribute for installation.
- Python 3.5 or above
- Program to open SVG objects (your browser should do it)
- Optional: Pillow to render barcodes as images (PNG, JPG, ...)
The best way is to use pip: pip install python-barcode
.
If you'll be exporting to images (eg: not just SVG), you'll need additional
optional dependencies, so run: pip install python-barcode[images]
.
- EAN-8
- EAN-13
- EAN-14
- UPC-A
- JAN
- ISBN-10
- ISBN-13
- ISSN
- Code 39
- Code 128
- PZN
- Add documentation
- Add more codes
Interactive:
>>> import barcode >>> barcode.PROVIDED_BARCODES ['code39', 'code128', 'ean', 'ean13', 'ean8', 'gs1', 'gtin', 'isbn', 'isbn10', 'isbn13', 'issn', 'jan', 'pzn', 'upc', 'upca'] >>> EAN = barcode.get_barcode_class('ean13') >>> EAN <class 'barcode.ean.EuropeanArticleNumber13'> >>> ean = EAN('5901234123457') >>> ean <barcode.ean.EuropeanArticleNumber13 object at 0x00BE98F0> >>> fullname = ean.save('ean13_barcode') >>> fullname 'ean13_barcode.svg' # Example with PNG >>> from barcode.writer import ImageWriter >>> ean = EAN('5901234123457', writer=ImageWriter()) >>> fullname = ean.save('ean13_barcode') 'ean13_barcode.png' # New in v0.4.2 >>> from io import BytesIO >>> fp = BytesIO() >>> ean.write(fp) # or >>> f = open('/my/new/file', 'wb') >>> ean.write(f) # Pillow (ImageWriter) produces RAW format here >>> from barcode import generate >>> name = generate('EAN13', '5901234123457', output='barcode_svg') >>> name 'barcode_svg.svg' # with file like object >>> fp = BytesIO() >>> generate('EAN13', '5901234123457', writer=ImageWriter(), output=fp) >>>
Now open ean13_barcode.[svg|png] in a graphic app or simply in your browser and see the created barcode. That's it.
Commandline:
$ python-barcode create "My Text" outfile New barcode saved as outfile.svg. $ python-barcode create -t png "My Text" outfile New barcode saved as outfile.png. Try `python-barcode -h` for help.
- Officially support Python 3.7
- Refer to Pillow in the docs, rather than PIL.
- Removed buggy
Barcode.raw
attribute. - Various CLI errors ironed out.
- Make the default value for
writer_options`
consistent across writers.
- Fix pushing of releases to GitHub.
- Fix crashes when attempting to use the CLI app.
- Properly include version numbers in SVG comments.
- Improve README rendering, and point to this fork's location (the outdated README on PyPI was causing some confusion).
- First release under the name
python-barcode
.
This project is a fork of pyBarcode, which, apparently, is no longer
maintained. v0.8.0 is our first release, and is the latest master
from that
parent project.
- Code 128 added.
- Data for charsets and bars moved to subpackage barcode.charsets.
- Merged in some improvements.
- Fixed some issues with fontsize and fontalignment.
- Added Python 3 support. It's not well tested yet, but the tests run without errors with Python 3.3. Commandline script added.
- Changed save and write methods to take the options as a dict not as keyword arguments (fix this in your code). Added option to left align the text under the barcode. Fixed bug with EAN13 generation.
- Added new generate function to do all generation in one step.
- Moved writer from a subpackage to a module (this breaks some existing code). UPC is now rendered as real UPC, not as EAN13 with the leading "0".
- Fixed bug in new write method (related to PIL) and updated docs.
- Added write method to support file like objects as target.
- Bugfix release. Removed redundancy in input validation.
- EAN8 was broken. It now works as expected.
- Removed **options from writers __init__ method. These options never had effect. They were always overwritten by default_options.
- New config option available: text_distance (the distance between barcode and text).
- Basic documentation included. The barcode object now has a new attribute called raw to have the rendered output without saving to disk.
- Support for rendering barcodes as images is implemented. PIL is required to use it.
- Compression for SVG output now works.
- Writer API has changed for simple adding new (own) writers.
- SVG output is now generated with xml.dom module instead of stringformatting (makes it more robust).
- API of render changed. Now render takes keyword arguments instead of a dict.
- More tests added.
- First release.