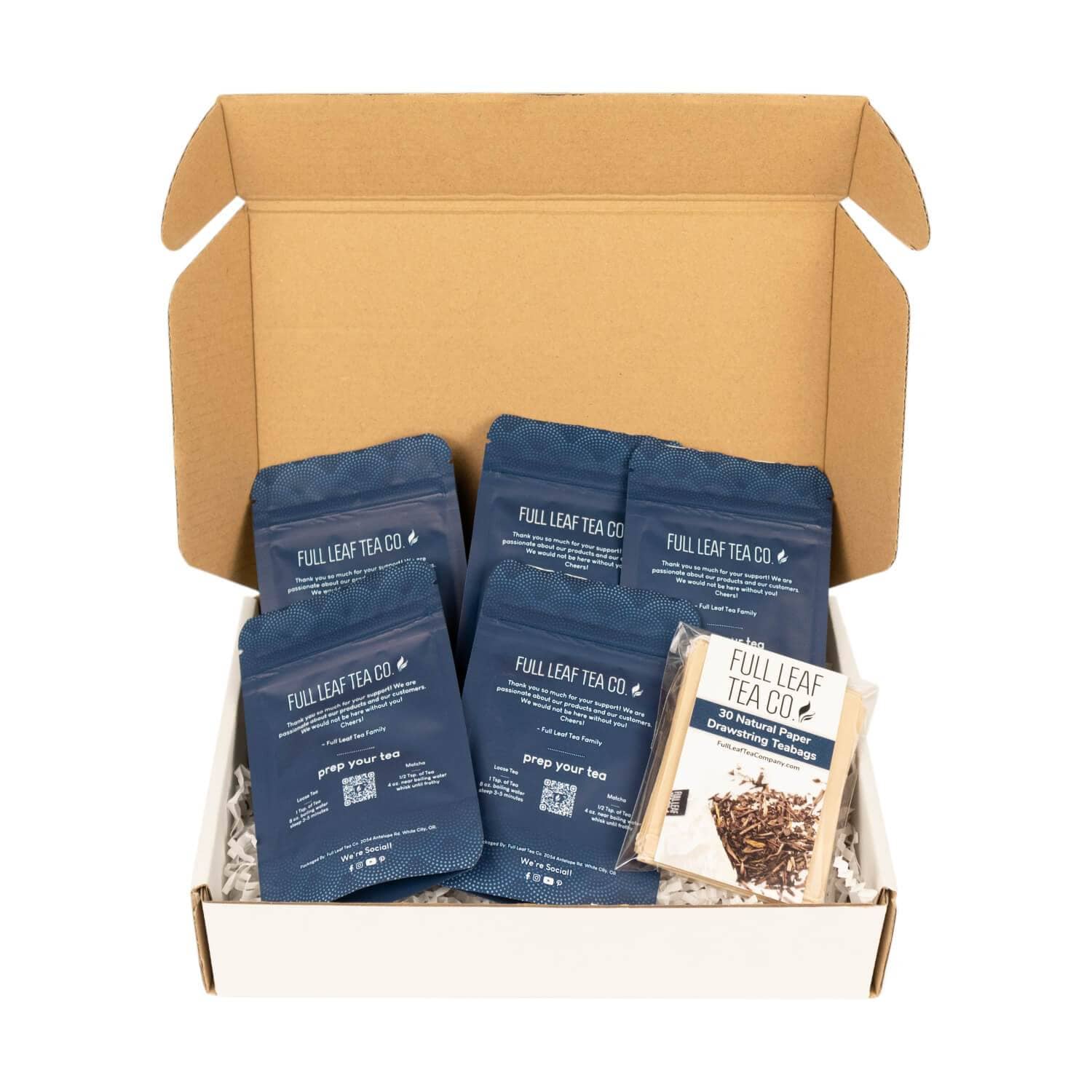
My Mod 4 take home challenge. An API application to help to manage subscriptions, customers, and teas for your tea company.
Report Bug
·
Request Feature
This is an API to manage customer subscriptions for a subscription tea service.
The Tea-scription API was developed as part of the curriculum at Turing School of Software and Design. The project aims to assess the following learning goals:
-
Strong Understanding of Rails: The project emphasizes the use of Rails, a popular web application framework, to build a robust API. By implementing this project, developers gain hands-on experience in utilizing Rails' powerful features and conventions.
-
Creating Restful Routes: Restful routes play a crucial role in designing well-structured APIs. In this project, developers are expected to create endpoints that follow RESTful principles, ensuring clarity, consistency, and ease of use for API consumers.
-
Demonstration of Well-Organized Code (OOP): Object-Oriented Programming (OOP) is a fundamental concept in software development. The project encourages developers to showcase their ability to write clean, organized, and maintainable code, leveraging OOP principles to enhance the structure and readability of their implementation.
-
Test-Driven Development (TDD): Test-driven development is an essential practice in modern software engineering. Throughout this project, developers are encouraged to follow TDD principles by writing tests before implementing the corresponding functionality. This approach ensures the reliability and stability of the API while facilitating code maintainability and extensibility.
-
Clear Documentation: Effective documentation is a vital aspect of any software project. By providing clear and comprehensive documentation, developers demonstrate their ability to communicate their work effectively to other developers and stakeholders. This project emphasizes the importance of documenting the API's endpoints, expected request/response formats, and any necessary setup steps.
Below you will see a list of prerequisites and steps to install and use this API.
You must have the following software installed on your system:
- Clone repository to local machine
- Install gems. Run
bundle install
in you console. - Create, migrate, and seed database by running
rails db:{create,migrate,seed}
in your console.
- After bundling, run
bundle exec rspec
to run test suite
- Run local server using
rails s
- Use postman/browser to reach endpoints described below
- Create a user subscription:
POST /api/v1/customers/:id/subscriptions
request: POST
/api/v1/customers/1/subscriptions w/ subscription params
response:
{
"data": {
"id": "10",
"type": "subscription",
"attributes": {
"title": "Green Tea Everyday",
"price": 14.5,
"frequency": "monthly",
"status": "active",
"tea_id": 1,
"customer_id": 1
}
}
}
- Update status of subscription:
PUT /api/v1/customers/:id/subscriptions/:id
request: PUT
/api/v1/customers/1/subscriptions/1
response:
{
"data": {
"id": "1",
"type": "subscription",
"attributes": {
"title": "Green Tea Everyday",
"price": 14.5,
"frequency": "weekly",
"status": "active",
"tea_id": 1,
"customer_id": 1
}
}
}
- Get all customer subecriptions (active & canceled):
GET /api/v1/customers/:id/subscriptions
request: GET
/api/v1/customers/1/subscriptions
response:
{
"data": [
{
"id": "1",
"type": "subscription",
"attributes": {
"title": "Green Tea Everyday",
"price": 14.5,
"frequency": "weekly",
"status": "cancelled",
"tea_id": 1,
"customer_id": 1
}
},
{
"id": "4",
"type": "subscription",
"attributes": {
"title": "Everyday Earl",
"price": 15.0,
"frequency": "weekly",
"status": "active",
"tea_id": 2,
"customer_id": 1
}
},
{
"id": "7",
"type": "subscription",
"attributes": {
"title": "Black Tea Pretty Frequently",
"price": 11.5,
"frequency": "biweekly",
"status": "active",
"tea_id": 3,
"customer_id": 1
}
}
]
}
Sam Walker – sgwalker327@gmail.com
https://github.com/sgwalker327/tea-scription
- Fork it (https://github.com/sgwalker327/tea-scription/fork)
- Create your feature branch (
git checkout -b feature/fooBar
) - Commit your changes (
git commit -am 'Add some fooBar'
) - Push to the branch (
git push origin feature/fooBar
) - Create a new Pull Request