javascript-helper-functions
Filter by some keyword in the entire array
var cities = [
{name: 'Los Angeles', population: '3799621',child: [{id: "child-1"}]},
{name: 'New York', population: '8175133',child: []},
{name: 'Old York', population: '1256938',child: [{id: "child-1"}]},
{name: 'Chicago', population: '2695598',child: [{id: "New York"}]},
{name: 'Houston', population: '2699451',child: [{id: "child-1"}]},
{name: 'Philadelphia', population: '1526006',child: [{id: "child-1"}]}
];
cities.filter(city => JSON.stringify(city).toUpperCase().includes('YoR'.toUpperCase()));
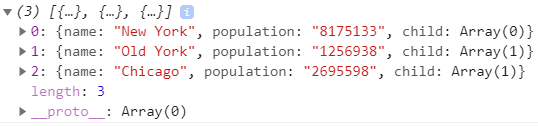
Get keys from Array and Object
let myArray = ['a', 'b', 'c'];
let arrayKeys = myArray.keys();
for(const key of arrayKeys) {
console.log(key);
}
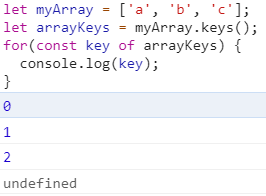
let myObject = {
a: 'string1',
b: 42,
c: 34
};
let myKeys = Object.keys(myObject);
console.log(myKeys);
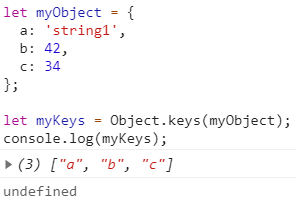