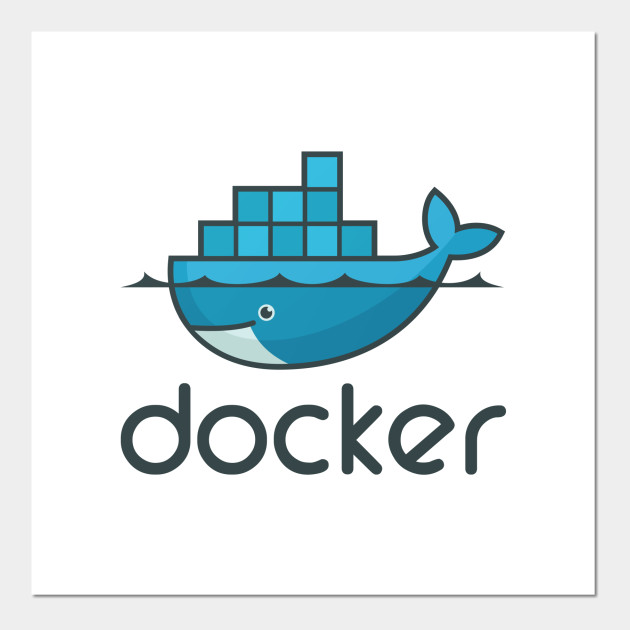
Docker is cool.
This is the exercise to build your muscle memory for docker commands. Type them in the terminal like a pro. It makes you look good. It makes you feel good. It makes everybody feel good. 🥳
This is for building docker command muscle memory. If you know these commands by heart, you can be the one who is saving the day when the production is down or helping your colleagues with their docker issues.
I hope this helps you to be the docker hero🤟💀🤟
Feel free to reach out to me🤙
Blog || Open Source Project || Band Camp
If you want to make a suggestion or contribute to this, feel free to pull the repo and make a pull request!
(1) Checking container stats
- (1) Check which container is using how much CPU or Memory.
- (2) Check the currently installed docker system information.
- (3) Inspect the container called node.
- (4) Check how Docker image's layer composition has changed over time and how recently.
- (5) See processes running on a container.
Answer
# (1) It displays a live stream of container resource usage statistics
docker stats
# (2)
docker info
# (3)
docker inspect node
# (4)
docker history <container name, e.g. node>
# (5)
docker top <CONTAINER ID>
(2) Streaming container log
Stream container log in the terminal.
Answer
-f will allow you to stream the log.docker logs -f <Container Name>
(3) Listing containers
List
- all the existing containers
- all the existing images
- all the running containers
Answer
docker container list --all
docker images -a
# or docker image ls
docker ps
(4) Pruning containers in the local machine
Remove the stopped container and all of the images, including unused or dangling images
Answer
docker system prune -a
(5) Runs commands in an already-running container.
Get into docker's container shell with interactive mode. Then exit.
Answer
docker exec
will allow you to execute a command against a running container. This is different from docker run
because docker run
will start a new container whereas docker exec
runs the command in an already-running container.
# -i: Interactive
# -t: Allocate a pseudo-TTY
docker exec -it <container-name> bash # this works most of the time
# some containers need to do it like this
docker exec -it <container-name> /bin/sh # e.g node alpine containers
docker exec -it <container-name> /bin/bash
# then exit
exit
(6) Run a react app from a local dist folder with nginx
Use nginx
container and map the local dist
folder to /usr/share/nginx/html
and server the react app.
Answer
docker run --mount type=bind,source="$(pwd)"/dist,target=/usr/share/nginx/html -p 8080:80 nginx
# or we could use volume...
docker run --volume "$(pwd)"/dist:/usr/share/nginx/html -p 8080:80 nginx
(1) Build and run from Dockerfile
Build a container with container name called my-app from the Dockerfile below with port mapping of 3000 to 3000. Then, restart the container.
FROM node:20-alpine
WORKDIR /app
COPY . .
RUN yarn install --production --frozen-lockfile
CMD ["node", "index.js"]
Answer
# Build the image
docker build -t my-app .
# Start the container with port mapped to 3000.
docker run -dp 3000:3000 my-app
# Stop the container
docker stop my-app
# Start the container
docker start my-app
(2) Running node app with node user
The above Dockerfile will run the app as a root user. Node containers have a user called node. Rewrite the file to run the app with the node user.
Answer
FROM node:20-alpine
# specify the user here
USER node
# use a node user directory.
WORKDIR /home/node/code
# change the ownership user -> node, group -> node
COPY --chown=node:node . .
RUN yarn install --production --frozen-lockfile
CMD ["node", "index.js"]
(3) Stop the container and remove
Stop the existing container named my-app and remove it.
Answer
# -f will stop and remove the container
docker rm <container-id or name> -f
# Alternatively stop and remove
docker stop <container-id or name>
docker rm <container-id or name>
(4) Avoid installing dependencies
Start a node app with node:alpine
container by node index.js
. Make sure that the dependencies are only installed when they are updated.
Answer
Docker automatically uses cache when there is no change in the files mentioned in the step. Each step is a layer and it can just use the cached layer when there is no change. Use --no-cache
option to rebuild everything from scratch.
FROM node:20-alpine
USER node
WORKDIR /home/node/code
COPY --chown=node:node yarn.lock package.json ./
RUN yarn install --production --frozen-lockfile
COPY --chown=node:node . .
CMD ["node", "index.js"]
(1) Starting all the existing containers
Starting all the existing container created by docker-compose.
Answer
docker-compose start
(2) Stop all the existing containers
Stopping all the existing container created by docker-compose.
Answer
docker-compose stop
(3) Rebuilding the containers
After updating docker file or docker-compose file, rebuild the containers.
Answer
This will update the container and restart the container. It will take up the terminal process. If you do ctrl
+ c
, it will stop all the containers.
docker-compose up --build
Of course, we can run container in a detached mode. This will keep all the container running.
docker-compose up --build -d
(4) Building one container from a particular docker-compose file
Build only app
service from docker-compose.ci.yml
Answer
We can just add the service name as blow. -f is to specify which file. -d is in detached mode.
docker-compose -f docker-compose.ci.yml up app -d