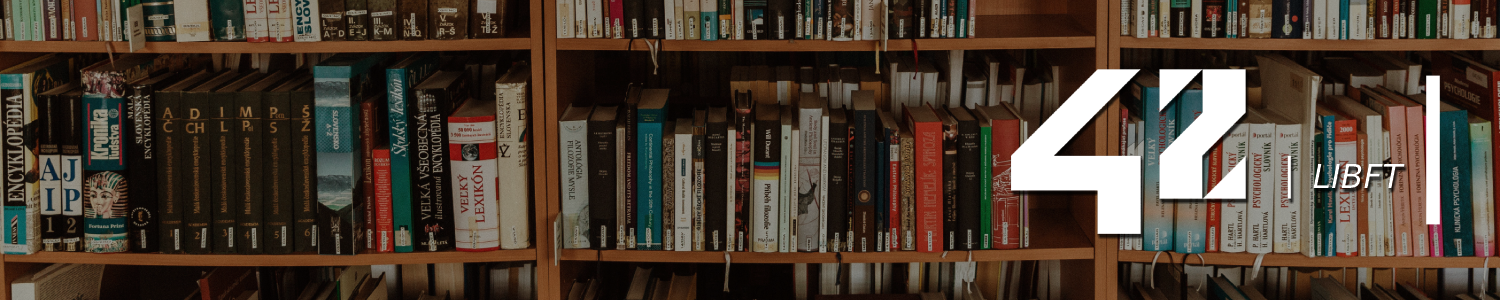
The first project of the 42 curriculum. It consists of recreating various standard functions of the language to be used during the course development, since we cannot use the originals. This is a living library and new functions can be added to it, increasing its functionalities. In total, forty-two functions were implemented, they are:
In your local repository
$ make
To use the library in your code you will need to include the header:
#include "libft.h"
When compiling your own code with libft
, don't forget to use the flags:
to create the objects
$ ... -I path/to/libft.h
to create the exe
$ ... -L path/to/libft.a -lft