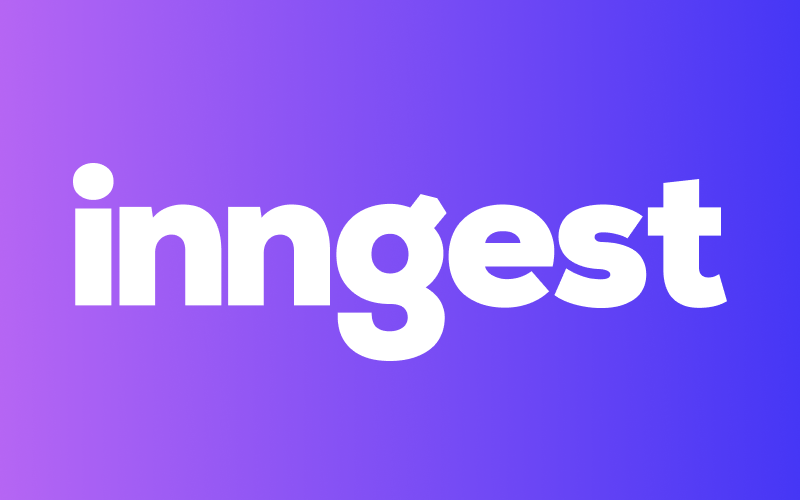
Serverless event-driven queues, background jobs, and scheduled jobs for Typescript.
Works with any framework and platform.
Inngest allows you to:
- ๐ Write background jobs in any framework, on any platform
- ๐ Create scheduled & cron jobs for any serverless platform
- ๐ Build serverless queues without configuring infra
- ๐ Write complex step functions anywhere
- ๐ Build serverless event-driven systems
- ๐ Reliably respond to webhooks, with retries & payloads stored for history
๐ Have a question or feature request? Join our Discord!
Getting started ยท Features ยท Contributing ยท Documentation
Install Inngest:
npm install inngest # or yarn install inngest
Writing functions: Write serverless functions and background jobs right in your own code:
import { createFunction } from "inngest";
export default createFunction(
"Send welcome email",
"app/user.created", // Subscribe to the `app/user.created` event.
({ event }) => {
sendEmailTo(event.data.id, "Welcome!");
}
);
Functions listen to events which can be triggered by API calls, webhooks, integrations, or external services. When a matching event is received, the serverless function runs automatically, with built in retries.
Triggering functions by events:
// Send events
import { Inngest } from "inngest";
const inngest = new Inngest({ name: "My App" });
// This will run the function above automatically, in the background
inngest.send("app/user.created", { data: { id: 123 } });
Events trigger any number of functions automatically, in parallel, in the background. Inngest also stores a history of all events for observability, testing, and replay.
- Fully serverless: Run background jobs, scheduled functions, and build event-driven systems without any servers, state, or setup
- Deploy anywhere: works with NextJS, Netlify, Vercel, Redwood, Express, Cloudflare, and Lambda
- Use your existing code: write functions within your current project, zero learning required
- A complete platform: complex functionality built in, such as event replay, canary deploys, version management and git integration
- Fully typed: Event schemas, versioning, and governance out of the box
- Observable: A full UI for managing and inspecting your functions
- Any language: Use our CLI to write functions using any language
Clone the repository, then:
yarn # install dependencies
yarn dev # build/lint/test
We use Volta to manage Node/Yarn versions.
When making a pull request, make sure to commit the changed
etc/inngest.api.md
file; this is a generated types/docs file that will highlight changes to the exposed API.
In order to provide sensible namespaced imports such as "inngest/next"
, the package actually builds to and deploys from dist/
.
To replicate this locally to test changes with other local repos, you can link the project like so (replace npm
for yarn
if desired):
# in this repo
yarn build
yarn prelink
cd dist/
yarn link
# in another repo
yarn link inngest